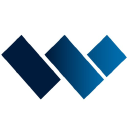
WorldCoinIndex
CryptocurrencyUsing Worldcoinindex Public API
Worldcoinindex is a platform that provides data and statistics concerning various cryptocurrencies. The Worldcoinindex API can be used for accessing cryptocurrency market statistics, exchange ticker data, and historical data. By using this API, developers can easily integrate cryptocurrency data into their applications.
Getting started
In order to start using the Worldcoinindex API, you need to do the following:
-
Create an account on the Worldcoinindex website and generate an API key.
-
Select the type of API you want to use: market data, ticker data or historical data.
-
Review the API documentation to select the relevant endpoints and parameters.
Market Data API
The Market Data API provides you with an overview of the cryptocurrency market. You can retrieve information such as the total market cap, total volume, and various market indexes.
Here's an example code in JavaScript:
const apiKey = 'your_api_key';
const apiUrl = 'https://www.worldcoinindex.com/apiservice/json?key=' + apiKey;
const endpoint = '/v2/stats';
fetch(apiUrl + endpoint)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we are using the Fetch API to send a GET request to the API endpoint. The response is received in JSON format, which we then log to the console.
Ticker Data API
The Ticker Data API provides real-time data regarding the prices, volumes, and trading pairs of cryptocurrencies across various exchanges.
Here's an example code in JavaScript:
const apiKey = 'your_api_key';
const apiUrl = 'https://www.worldcoinindex.com/apiservice/json?key=' + apiKey;
const endpoint = '/ticker';
fetch(apiUrl + endpoint)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we are fetching ticker data for all cryptocurrencies. However, you can also specify the cryptocurrency and exchange to get specific ticker data.
Historical Data API
The Historical Data API allows you to fetch historical data for various cryptocurrencies. You can get price data as well as volume data.
Here's an example code in JavaScript:
const apiKey = 'your_api_key';
const apiUrl = 'https://www.worldcoinindex.com/apiservice/json?key=' + apiKey;
const endpoint = '/history';
const cryptocurrency = 'BTC';
const currency = 'USD';
const period = '1month';
fetch(apiUrl + endpoint + '/' + cryptocurrency + '/' + currency + '/' + period)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In the above code, we are fetching historical data for the Bitcoin cryptocurrency in USD currency for a period of one month.
Conclusion
The Worldcoinindex API is a powerful tool for accessing cryptocurrency data. You can use it to retrieve market data, ticker data, or historical data. By using this API, you can easily integrate cryptocurrency data into your application.