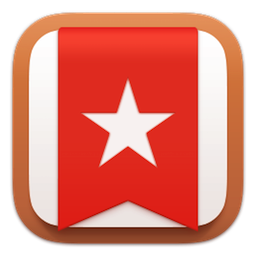
Wunderlist
Documents & ProductivityExploring the Wunderlist Public API
Wunderlist is a task and to-do list management application that offers a public API for developers to interface with their platform. The API is free to use and provides access to the full functionality of the Wunderlist platform.
Overview of the API
The Wunderlist API provides developers with four endpoints to interact with the platform; lists, tasks, notes, and reminders. Each endpoint offers a range of methods to create, read, update, and delete data on the Wunderlist platform.
The documentation for the Wunderlist API is thorough and well-written. The API reference provides examples of each method call along with the parameters required to perform the call. Additionally, the documentation offers guides for common use cases on the Wunderlist platform.
Authenticating with the API
Before making any API calls, you'll need to authenticate with the Wunderlist platform. To do so, you'll need to create an application on the Wunderlist developer portal. Once you've created your application, you'll receive a client ID and client secret, which you can use to issue OAuth tokens to your users.
Here's an example in JavaScript of how to authenticate with the Wunderlist platform:
const OAUTH_URL = "https://www.wunderlist.com/oauth/authorize";
const CLIENT_ID = "YOUR_CLIENT_ID";
function authenticate() {
const redirectUrl = `${window.location.origin}/oauth/callback`;
const authUrl = `${OAUTH_URL}?client_id=${CLIENT_ID}&redirect_uri=${redirectUrl}`;
window.location.href = authUrl;
}
Getting a List of Lists
Once you've authenticated with the Wunderlist platform, you can start making API calls. The first call you might want to make is to get a list of lists. Here's how you can do that in JavaScript:
const LIST_API = "https://a.wunderlist.com/api/v1/lists";
async function getLists(accessToken) {
const response = await fetch(LIST_API, {
method: "GET",
headers: {
"X-Access-Token": accessToken,
"X-Client-ID": CLIENT_ID,
},
});
const data = await response.json();
return data;
}
This function will make a GET request to the Wunderlist API to fetch a list of all lists associated with the authenticated user's account. The function expects an access token, which you can obtain through the OAuth flow.
Creating a New Task
Once you have a list of lists, you can start working with tasks. Here's an example of how to create a new task on a list in JavaScript:
async function createTask(accessToken, listId, title) {
const response = await fetch(`${LIST_API}/${listId}/tasks`, {
method: "POST",
headers: {
"Content-Type": "application/json",
"X-Access-Token": accessToken,
"X-Client-ID": CLIENT_ID,
},
body: JSON.stringify({ title }),
});
const data = await response.json();
return data;
}
To create a new task, you need to issue a POST request to the "tasks" endpoint on the list you want to add the task to.
Updating a Task
Once you've created a task, you might want to update its details. Here's how you can do that in JavaScript:
async function updateTask(accessToken, taskId, title) {
const response = await fetch(`${TASK_API}/${taskId}`, {
method: "PATCH",
headers: {
"Content-Type": "application/json",
"X-Access-Token": accessToken,
"X-Client-ID": CLIENT_ID,
},
body: JSON.stringify({ title }),
});
const data = await response.json();
return data;
}
This function will issue a PATCH request to update the task's details.
Conclusion
The Wunderlist public API provides developers with a powerful tool to interact with the platform. By utilizing the example code provided in this article, you can begin building your own applications that leverage the features of the Wunderlist platform.