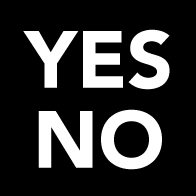
Yes No
DevelopmentGenerate yes or no randomly. An example JSON response randomly generated on this link :- {"answer":"no","forced":false,"image":"https://yesno.wtf/assets/no/7-331da2464250a1459cd7d41715e1f67d.gif"}
π Documentation & Examples
Everything you need to integrate with Yes No
π Quick Start Examples
// Yes No API Example
const response = await fetch('https://yesno.wtf/api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the YesNo API in JavaScript
The YesNo API is a simple and fun API that allows you to get a random "yes" or "no" answer to any question you may have. It's free to use, and requires no authentication or API key.
In this blog post, we will be exploring how to use the YesNo API in JavaScript, with some example code.
Example API requests
To get started, let's take a look at some example API requests:
Get a random answer
fetch('https://yesno.wtf/api')
.then(response => response.json())
.then(data => console.log(data.answer));
This code uses the fetch
function to send a GET request to the API endpoint. Once the response is received, we parse the JSON data and log the answer to the console.
Get a specific answer
fetch('https://yesno.wtf/api?force=maybe')
.then(response => response.json())
.then(data => console.log(data.answer));
In this example, we add a query parameter force
to the API request with a value of "maybe". This will result in a response with an answer of "maybe".
Get a GIF instead of a text answer
fetch('https://yesno.wtf/api?gif=true')
.then(response => response.json())
.then(data => console.log(data.image));
By adding the query parameter gif
with a value of "true", we can get a GIF instead of a text answer. The response will include a URL to a GIF representing the answer.
Conclusion
Using the YesNo API in JavaScript is simple and straightforward. With just a few lines of code, you can get a random "yes" or "no" answer to any question, as well as add some fun customization with query parameters.
π 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes