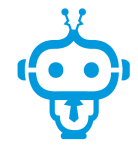
Zip Code Data
GeocodingA Guide to Using the Zip Code API by MetaDapi
MetaDapi's Zip Code API is a powerful tool that allows developers to retrieve valuable information based on zip codes. With this API, you can quickly and easily access data related to location, population, demographics, weather, and more. In this blog post, we'll explore how to use the Zip Code API and provide several examples in JavaScript.
Getting Started
Before we dive into the code, there are a few things you'll need to do to get started with the Zip Code API. First, you'll need to sign up for an account on the MetaDapi website and acquire an API key. Once you have your API key, you can start making requests to the API.
The API request will return a JSON object containing all of the information associated with the provided zip code.
Example 1: Get the City and State Based on a Zip Code
const zipcode = "90210";
const apiKey = "YOUR_API_KEY_HERE";
const url = `https://www.metadapi.com/api/v1/zip/${zipcode}?apikey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data.city, data.state));
In this example, we provide "90210" as the zip code and replace "YOUR_API_KEY_HERE" with the actual API key. The fetch function retrieves the data from the API endpoint, and we can then access the city and state values in the returned JSON object.
Example 2: Get the Weather Information Based on a Zip Code
const zipcode = "90210";
const apiKey = "YOUR_API_KEY_HERE";
const url = `https://www.metadapi.com/api/v1/zip/${zipcode}/weather?apikey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
In this example, we add "/weather" to the API endpoint and retrieve weather information based on the specified zip code. The returned JSON object contains a variety of weather-related data, including the current temperature, wind speed, humidity, and more.
Example 3: Get the Population Information Based on a Zip Code
const zipcode = "90210";
const apiKey = "YOUR_API_KEY_HERE";
const url = `https://www.metadapi.com/api/v1/zip/${zipcode}/demographics?apikey=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data.population));
In this final example, we add "/demographics" to the API endpoint and retrieve population information based on the specified zip code. The returned JSON object contains a variety of demographic data, including population information broken down by age, gender, and ethnicity.
Conclusion
Overall, the Zip Code API by MetaDapi is a powerful tool that provides access to a wealth of data related to zip codes. Whether you're looking to retrieve weather data, demographic information, or something else entirely, this API is a great resource for developers looking to build powerful applications. With the examples provided in this blog post, you should now have a good understanding of how to use the Zip Code API in JavaScript.