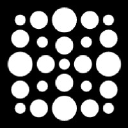
ZMOK
CryptocurrencyThe Ethereum JSON RPC API is a powerful interface that allows developers to interact seamlessly with the Ethereum blockchain. This API serves as a vital bridge for accessing blockchain data, executing smart contracts, and monitoring transactions in real-time. With its Web3 provider capabilities, users can integrate Ethereum functionalities into their applications with ease, enabling quick deployment of decentralized applications (dApps). By utilizing the Ethereum JSON RPC API, developers gain the ability to harness the full potential of Ethereum's network, providing valuable services and features to end users while ensuring optimal performance and security.
Using the Ethereum JSON RPC API comes with numerous advantages that elevate the development experience. Key benefits include:
- Seamless integration with dApps using familiar JavaScript syntax.
- Comprehensive access to Ethereum blockchain data and smart contract functionalities.
- Support for a wide range of Ethereum network operations, from sending transactions to querying contract events.
- High reliability and performance for real-time data retrieval and command execution.
- Active community and documentation support, ensuring developers have the resources they need.
Here's a simple JavaScript example demonstrating how to call the Ethereum JSON RPC API:
const Web3 = require('web3');
// Set up a Web3 provider using Infura or any Ethereum node
const web3 = new Web3(new Web3.providers.HttpProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID'));
// Example function to get the current block number
async function getCurrentBlock() {
try {
const blockNumber = await web3.eth.getBlockNumber();
console.log(`Current Ethereum Block Number: ${blockNumber}`);
} catch (error) {
console.error('Error fetching block number:', error);
}
}
// Call the function
getCurrentBlock();