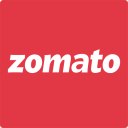
Zomato
Food & DrinkGetting Started with Zomato Public API
If you're a developer looking to integrate restaurant data into your application, Zomato Public API might be what you're looking for. Zomato is an online restaurant search and discovery platform that provides detailed information about restaurants, including menus, photos, user reviews, and more. Their API allows developers to access this data programmatically and build their applications around it. In this blog post, we'll explore how to use Zomato Public API by providing some JavaScript examples.
Creating an Account and Accessing the API
Before using Zomato Public API, you need to sign up for an account on their website and get your API key. Once you have your API key, you can make API requests to the Zomato server via HTTP requests.
Example of HTTP Request
Here's an example of an HTTP request to the Zomato API endpoint to get a list of restaurants in a specific location.
const apiKey = 'your_api_key_here';
const location = 'Toronto';
//Set headers
const headers = new Headers({
'Content-Type': 'application/json',
'user-key': apiKey
});
//Make a GET request to the API with a location parameter
fetch(`https://developers.zomato.com/api/v2.1/locations?query=${location}`, { headers })
.then(response => response.json())
.then(data => {
const locationID = data.location_suggestions[0].entity_id;
console.log(`Location ID for ${location}: ${locationID}`);
// Make a GET request to the restaurant search endpoint with location id parameter
return fetch(`https://developers.zomato.com/api/v2.1/search?entity_id=${locationID}&entity_type=city`, { headers });
})
.then(response => response.json())
.then(data => {
console.log(data.restaurants);
})
.catch(error => console.error(error));
In this example, we are using the fetch
function in JavaScript to make an HTTP GET request to the Zomato API with the location
parameter. The response is a JSON object containing the location's ID, which can be used in another API request to get a list of restaurants in that location.
Example API Call
Here's an example of how to retrieve information about a specific restaurant using its ID.
const apiKey = 'your_api_key_here';
const restaurantID = '16507624';
// Set headers
const headers = new Headers({
'Content-Type': 'application/json',
'user-key': apiKey
});
// Make a GET request to the restaurant endpoint with restaurant id parameter
fetch(`https://developers.zomato.com/api/v2.1/restaurant?res_id=${restaurantID}`, { headers })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we are using the fetch
function in JavaScript to make an HTTP GET request to the Zomato API with the restaurantID
parameter. The response is a JSON object containing detailed information about the restaurant.
Conclusion
This blog post provides some examples of how to use Zomato Public API. However, this is just the tip of the iceberg. Zomato Public API offers many more endpoints and parameters that allow developers to retrieve and manipulate restaurant data programmatically. If you're interested in exploring more, head over to their website and explore the documentation. Happy coding!