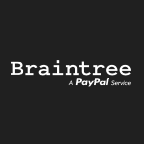
Braintree
FinanceAccept and process cards, wallets, and Local Payment Methods in a single, modern integration. Store customer and payment information to reduce friction at checkout. Build a payment form with the right level of customization for your needs. Add payment methods your customers want to your checkout. Securely store customer information – including payment method information.
📚 Documentation & Examples
Everything you need to integrate with Braintree
🚀 Quick Start Examples
// Braintree API Example
const response = await fetch('https://developers.braintreepayments.com/?ref=apilist.fun', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
BrainTree Payments API - A Comprehensive Overview
If you're in the market for a payments processor, then look no further than BrainTree Payments. It's a platform that allows businesses to manage all their payments in one centralized location. And, it provides an API that lets developers customize payment processing to fit their specific needs.
Here's a step-by-step guide that will take you through everything you need to know to integrate the BrainTree Payments API with your website or mobile application using JavaScript.
1. Sign Up and Get Your API Keys
To get started with the BrainTree Payments API, you'll first need to sign up for a developer account. Once you have your account, you can generate your API credentials by navigating to the API Keys section of the dashboard.
2. Include the JavaScript Library
The first thing you'll need to do is include the BrainTree JavaScript library on your site. You can do this by adding the following script tag to your HTML file:
<script src="https://js.braintreegateway.com/web/3.78.0/js/client.min.js"></script>
3. Initialize the Client
To use the API, you'll first need to create a new instance of the client object using your API keys, like so:
const client = new braintree.api.Client({
authorization: 'your_api_key_here'
});
4. Create a Payment Method
To create a new payment method, you'll need to obtain a payment-method nonce. The payment-method nonce is a unique identifier that represents the payment method data collected by the Braintree client SDKs. Here's an example of how to create a new hosted fields payment form and obtain the payment-method nonce:
braintree.hostedFields.create({
client: client,
fields: {
number: '#card-number',
cvv: '#cvv',
expirationDate: '#expiration-date',
postalCode: '#postal-code',
cardholderName: '#cardholder-name'
}
}, function (err, hostedFieldsInstance) {
if (err) {
console.error(err);
return;
}
// Submit the payment form
hostedFieldsInstance.tokenize(function (err, payload) {
if (err) {
console.error(err);
return;
}
// The nonce is the key to using the BrainTree Payments API. You can use it to
// create a transaction, add a payment method to a customer, or do anything else
// that requires a payment method.
const nonce = payload.nonce;
console.log(nonce);
});
});
5. Create a Transaction
Once you have a payment-method nonce, you can use it to create a transaction. Here's an example of how to create a new transaction:
// Create a new transaction request
const request = {
amount: '10.00',
paymentMethodNonce: nonce,
options: {
submitForSettlement: true
}
};
// Submit the transaction request
client.request({
endpoint: 'transactions',
method: 'post',
data: request
}, function(err, response) {
if (err) {
console.error(err);
return;
}
console.log(response);
});
That's all there is to it! With these simple steps, you can integrate the BrainTree Payments API with your website or mobile application using JavaScript. Happy coding!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes