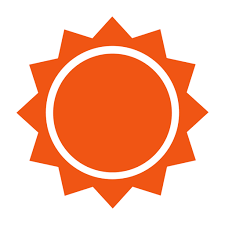
AccuWeather
WeatherThe AccuWeather APIs, available on their developer website, provide a range of weather and forecast data that can be integrated into various applications and services. With these APIs, developers can access current weather conditions, hourly and daily forecasts, and historical weather data for locations around the world. The AccuWeather APIs are powered by advanced forecasting technologies, providing accurate and reliable weather information to millions of people every day. Whether you're building a weather app, a travel website, or just want to stay informed about the latest weather conditions, the AccuWeather APIs are a valuable resource that can help you meet your needs. So why not check them out today and see how they can enhance your applications and services?
📚 Documentation & Examples
Everything you need to integrate with AccuWeather
🚀 Quick Start Examples
// AccuWeather API Example
const response = await fetch('https://developer.accuweather.com/apis', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
AccuWeather Public API
AccuWeather offers a range of public APIs for developers. These APIs enable developers to access various weather-related data like temperature, humidity, wind speed, etc. In this article, we will explore some of the API examples in JavaScript.
To use the AccuWeather APIs, you need an API key. You can get a free API key by registering at the AccuWeather Developer Website. Once you have an API key, you can use it to make requests to the AccuWeather APIs.
API Example 1: Get Current Weather Conditions
To get the current weather conditions for a specific location, you can use the Current Conditions API. The locationKey
in the API endpoint is a unique identifier for a specific location.
Here's an example code in JavaScript:
const apiKey = 'YOUR_API_KEY';
const locationKey = 'LOCATION_KEY';
const apiUrl = `http://dataservice.accuweather.com/currentconditions/v1/${locationKey}?apikey=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
console.log(data);
// Do something with the data
})
.catch(error => console.error(error));
API Example 2: Get Hourly Weather Forecast
To get the hourly weather forecast for a specific location, you can use the Hourly Forecast API. The locationKey
in the API endpoint is a unique identifier for a specific location.
Here's an example code in JavaScript:
const apiKey = 'YOUR_API_KEY';
const locationKey = 'LOCATION_KEY';
const apiUrl = `http://dataservice.accuweather.com/forecasts/v1/hourly/${locationKey}?apikey=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
console.log(data);
// Do something with the data
})
.catch(error => console.error(error));
API Example 3: Get Daily Weather Forecast
To get the daily weather forecast for a specific location, you can use the Daily Forecast API. The locationKey
in the API endpoint is a unique identifier for a specific location, and the period
is the number of days in the forecast, up to 5 days.
Here's an example code in JavaScript:
const apiKey = 'YOUR_API_KEY';
const locationKey = 'LOCATION_KEY';
const period = 5;
const apiUrl = `http://dataservice.accuweather.com/forecasts/v1/daily/${period}day/${locationKey}?apikey=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
console.log(data);
// Do something with the data
})
.catch(error => console.error(error));
Conclusion
AccuWeather offers a range of public APIs that can be used to access weather-related data for various locations. We explored some of the examples using JavaScript, but you can use any programming language that supports HTTP requests to work with these APIs. With these APIs, you can create applications that can provide accurate weather forecasts to your users.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes