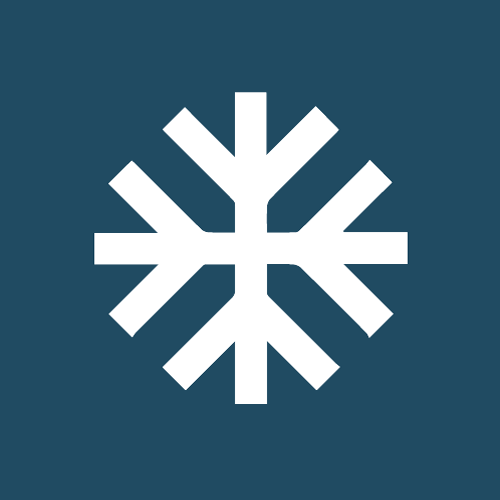
APIXU
WeatherAPIXU weather and geolocation API provides you with access to get real-time, future and historical data ranging from weather, sports, astronomy, currency and much more for your website or application for any IP address, city, zip or geo point in the world. Real-Time & Historical World Weather Data API Retrieve instant, accurate weather information for any location in the world in lightweight JSON format Trusted by 75,000 companies worldwide START USING THE API — It's free!
📚 Documentation & Examples
Everything you need to integrate with APIXU
🚀 Quick Start Examples
// APIXU API Example
const response = await fetch('https://www.apixu.com/doc/request.aspx', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using the Apixu API with JavaScript
The Apixu API is a great tool for accessing weather data. It covers a wide range of weather parameters across the globe. In this article, we'll show you how to use the API with JavaScript.
Getting Started
To get started, you'll need an API key. You can get a free API key by registering on the Apixu website.
Once you have your API key, you can start making requests. The easiest way to do this is by sending a GET request using XHR or fetch.
XHR Example
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://api.apixu.com/v1/current.json?key=YOUR_API_KEY&q=Paris');
xhr.onload = function() {
if (xhr.status === 200) {
const data = JSON.parse(xhr.responseText);
console.log(data);
} else {
console.log('Error');
}
};
xhr.send();
Fetch Example
fetch('https://api.apixu.com/v1/current.json?key=YOUR_API_KEY&q=Paris')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
Using the API
The Apixu API has a wide range of parameters that you can use to get specific weather data for a location. Here are a few examples.
Current Weather
fetch('https://api.apixu.com/v1/current.json?key=YOUR_API_KEY&q=Paris')
.then(response => response.json())
.then(data => console.log(data.current))
.catch(error => console.log(error));
Forecast
fetch('https://api.apixu.com/v1/forecast.json?key=YOUR_API_KEY&q=Paris')
.then(response => response.json())
.then(data => console.log(data.forecast))
.catch(error => console.log(error));
History
fetch('https://api.apixu.com/v1/history.json?key=YOUR_API_KEY&q=Paris&dt=2019-04-01')
.then(response => response.json())
.then(data => console.log(data.forecast))
.catch(error => console.log(error));
Conclusion
The Apixu API is a powerful tool for getting detailed weather data. By using XHR or fetch, we can easily integrate it into our JavaScript projects. With the wide range of parameters available, we can customize our API requests to get exactly the data we need.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes