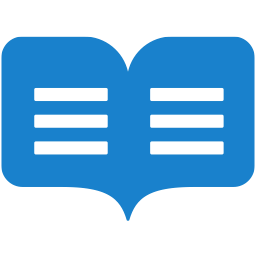
Archive.org
Open DataThe Internet Archive is a nonprofit organization committed to Universal Access of Knowledge. The Archive runs several services including the Archive.org search engine, OpenLibrary, and the Wayback Machine. In alignment with its mission, the Archive encourages developers to add media to archive.org, as well as to consume and re-purpose media and its metadata, for the great good of our community and beyond.
📚 Documentation & Examples
Everything you need to integrate with Archive.org
🚀 Quick Start Examples
// Archive.org API Example
const response = await fetch('https://archive.readme.io/docs', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the Archive API using JavaScript
The Archive API allows you to search through archival materials and retrieve information on various historical collections. With a simple use of HTTP requests, you can easily access the data and make use of it in your applications.
Here are a few examples of how to access the Archive API in JavaScript.
Basic API Usage
To get started, you will first need to acquire an API key from the Archive website. Once you have this, you can use the following JavaScript code to make requests to the API, using the fetch
method to send a GET request:
const apiKey = 'INSERT API KEY HERE';
const apiBase = 'https://archive.readme.io/api';
const endpoint = 'collections/search?q=harry+potter';
fetch(apiBase + endpoint, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we are searching for collections related to Harry Potter. Make sure to replace INSERT API KEY HERE
with your actual API key.
Filtering Data
To narrow down your search results, you can add query parameters to your GET requests. For example, to search for collections related to Harry Potter that also contain the term "illustrated", you can use the following code:
const apiKey = 'INSERT API KEY HERE';
const apiBase = 'https://archive.readme.io/api';
const endpoint = 'collections/search?q=harry+potter&filter=illustrated';
fetch(apiBase + endpoint, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we have added the filter
parameter to the endpoint URL to search for collections that contain "illustrated".
Retrieving Specific Collections
To retrieve specific collections from the API, you can use the id
parameter in your requests. For example, to retrieve the details for a collection with the ID MyCollection123
, you can use the following code:
const apiKey = 'INSERT API KEY HERE';
const apiBase = 'https://archive.readme.io/api';
const endpoint = 'collections/MyCollection123';
fetch(apiBase + endpoint, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data));
In this example, we are using the collections/MyCollection123
endpoint to retrieve the details for the collection with the ID of MyCollection123
.
These are just a few examples of how to use the Archive API in your JavaScript applications. To learn more about the API and its capabilities, check out the full documentation at https://archive.readme.io/docs.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes