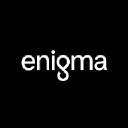
Enigma Public
Open DataEnigma's API offers the broadest collection of public data, enabling developers and businesses to unlock valuable insights from diverse datasets. With its comprehensive access to public information, users can easily integrate, analyze, and visualize data in their applications. This powerful API not only streamlines the process of accessing vast amounts of public data but also enhances the capability to make data-driven decisions. By leveraging this resource, organizations can uncover trends, enrich their datasets, and drive innovation in their solutions, ultimately transforming the way they operate in today's data-centric world.
Using Enigma's API comes with numerous benefits that enhance your development process and data strategy. These include improved access to diverse datasets, increased efficiency for data retrieval, scalability to handle large data requests, support for various data formats, and robust documentation that simplifies integration. The following points summarize the key advantages of utilizing this API:
- Access to the largest collection of public datasets
- Rapid data retrieval to save time and resources
- Scalable architecture to meet growing data needs
- Compatibility with multiple programming languages and formats
- Comprehensive documentation and support for developers
Here’s a JavaScript code example for calling the Enigma API:
const axios = require('axios');
const fetchPublicData = async () => {
try {
const response = await axios.get('https://api.enigma.com/v1/data', {
headers: {
'Authorization': 'Bearer YOUR_API_TOKEN'
}
});
console.log(response.data);
} catch (error) {
console.error('Error fetching data:', error);
}
};
fetchPublicData();