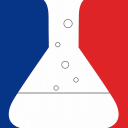
French Address Search
Open DataThe Address Search API provided by the French Government is a powerful tool for developers looking to integrate reliable address verification and geolocation features into their applications. By leveraging this API, businesses can enhance user experience by ensuring accurate address input and validation, which can ultimately lead to improved operational efficiency and better customer service. The API enables users to search for addresses across France by providing a comprehensive dataset that includes postal codes, streets, and coordinates, making it an essential resource for e-commerce platforms, local services, and any application requiring address-related features.
Utilizing the French Government's Address Search API comes with several advantages. Here are five key benefits of using this API:
- Access to official government data, ensuring accuracy and reliability.
- Enhanced user experience through real-time address suggestions and autocomplete functionality.
- Ability to retrieve geographic coordinates for addresses, enabling effective mapping solutions.
- Support for a wide range of search functionalities, including filtering options based on various parameters.
- Free access with high availability, making it an economical choice for developers.
Here's a JavaScript code example demonstrating how to call the Address Search API:
const fetch = require('node-fetch');
async function searchAddress(query) {
const url = `https://api-adresse.data.gouv.fr/search/?q=${encodeURIComponent(query)}`;
try {
const response = await fetch(url);
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching address data:', error);
}
}
// Example usage
searchAddress("10 rue de la Paix, Paris");