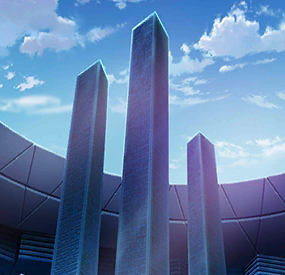
Atlas Academy
Games & ComicsExploring the Atlas Academy API Using JavaScript
Atlas Academy is a popular API platform that provides comprehensive documentation and tools for developers to access a variety of data sources, such as Japanese mobile game Fate/Grand Order.
In this article, we will cover how to utilize the Atlas Academy API to retrieve data and combine it with JavaScript. Below is an example of using Atlas Academy API to retrieve the stats of certain servants:
const fetch = require('node-fetch');
fetch('https://api.atlasacademy.io/nice/JP/servant/1/stats')
.then(response => response.json())
.then(data => console.log(data));
Here we are using the Node.js 'node-fetch' module to send a GET request to the Atlas Academy API. We pass in the endpoint URL, which in this case is used to retrieve the stats of the first Japanese servant in Fate/Grand Order.
After receiving the response, we parse the JSON data and log it to the console. This code will produce the following output:
{
"id":1,
"name":"アルトリア・ペンドラゴン",
"classType":"SABER",
"rarity":5,
"cost":16,
"lvMax":90,
"atkMax":12994,
"hpMax":17328,
"atkBase":1868,
"hpBase":2994
}
Another useful endpoint in Atlas Academy is the 'search/' endpoint. It allows us to retrieve data that contains the specified search keywords. The following example demonstrates how to retrieve all servant data that matches the search keyword 'saber':
const fetch = require('node-fetch');
fetch('https://api.atlasacademy.io/nice/JP/search/?name=saber&type=servant')
.then(response => response.json())
.then(data => console.log(data));
We first pass the search keyword into the 'name' parameter and the specified data type into the 'type' parameter. Here we are searching for servant data that contains 'saber' in its name.
We then parse the JSON response and log it to the console. The resulting output will contain information about all servants that match the search criteria.
Lastly, we can also use the Atlas Academy API to retrieve NP data. Here is an example of retrieving the NP data for a specific servant:
const fetch = require('node-fetch');
fetch('https://api.atlasacademy.io/nice/JP/servant/noble-phantasm/1')
.then(response => response.json())
.then(data => console.log(data));
This code retrieves the NP data of the first servant in the Japanese version of Fate/Grand Order. After parsing the JSON response, we will get the following output:
{
"id":1,
"name":"約束された勝利の剣(エクスカリバー)",
"cardType":"BUSTER",
"rank":"B+",
"detail":"魔力放出の総量を極限にまで高めて放つ宝具。実体剣として現れた英霊が放つ必滅の剣。",
"effect":"敵全体に攻撃(威力:900%〜1500%)、自身のHPを回復[自身のMaxHPの割合:50]。",
"hit":1
}
In conclusion, the Atlas Academy API provides an excellent resource for accessing a wide variety of data sources that are useful for building applications and retrieving vital game information. Utilizing JavaScript with these APIs opens up a whole new world of possibilities for game developers and designers alike.