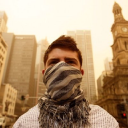
📚 Documentation & Examples
Everything you need to integrate with Steam
🚀 Quick Start Examples
// Steam API Example
const response = await fetch('https://steamapi.xpaw.me/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Steam Web API is a powerful set of HTTP interfaces that allows you to access a range of valuable data related to gaming on the popular platform, Steam. This API gives developers a powerful tool to integrate and manipulate various gaming functionalities, stats, and data into their applications or websites. Steam Web API provides a well-structured data collection, from user profiles, owned games, and friends list, to achievements, leaderboards, and gameplay stats, offering rich data possibilities for any developer working on gaming related projects.
Steam Web API's documentation, which is available here, provides a detailed guide on the different endpoints available, response formats, and how to successfully call the API. The document gives clear instructions and examples, making it easy even for beginners to implement and use it.
The benefits of using Steam Web API include:
-
Access to rich gaming-related data including user profiles and gameplay statistics.
-
Provides real-time data which can be very useful for real-time applications.
-
The API is robust, reliable, and continuously updated, making it a trustworthy data source for professional applications.
-
Opportunity to use gaming data to provide personalized experiences to users.
-
Easy to use with clear documentation, examples, and support, helping both new and experienced developers.
Here's a sample JavaScript code for calling the Steam Web API:
var xhr = new XMLHttpRequest();
var key = 'YOUR_STEAM_API_KEY';
var steamid = 'TARGET_STEAM_ID';
xhr.open("GET", `http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key=${key}&steamids=${steamid}`, true);
xhr.onreadystatechange = function (){
if(xhr.readyState == 4 && xhr.status == 200)
console.log(JSON.parse(xhr.responseText));
}
xhr.send();
In this example, make sure to replace 'YOUR_STEAM_API_KEY'
and 'TARGET_STEAM_ID'
with your API key and the targeted Steam ID respectively.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes