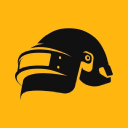
PUBG
Games & ComicsThe PUBG API offers developers seamless access to a wealth of in-game data from PLAYERUNKNOWN'S BATTLEGROUNDS, enabling integration of live gameplay statistics, player profiles, and match histories into various applications. By leveraging this robust API, developers can provide real-time insights, track player performance, and enhance user engagement through data-driven features. With comprehensive documentation available at developer.pubg.com, developers can easily navigate and utilize the API to build innovative gaming applications, tools, and services that resonate with PUBG's vast player community.
Using the PUBG API comes with numerous advantages, including:
- Real-time access to player statistics and match history
- Ability to track competitive performance and rankings
- Integration capabilities for enhanced user experience across platforms
- Access to event data, helping users stay informed about ongoing tournaments
- Support for community engagement through detailed player insights and achievements
Here’s a simple JavaScript code example demonstrating how to call the PUBG API:
const fetch = require('node-fetch');
const API_KEY = 'YOUR_PUBG_API_KEY';
const PLAYER_NAME = 'sample_player_name';
async function getPlayerData() {
const url = `https://api.pubg.com/shards/steam/players?filter[playerNames]=${PLAYER_NAME}`;
try {
const response = await fetch(url, {
method: 'GET',
headers: {
'Accept': 'application/json',
'Authorization': `Bearer ${API_KEY}`
}
});
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('There has been a problem with your fetch operation:', error);
}
}
getPlayerData();