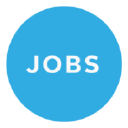
Authentic Jobs
JobsAuthentic Jobs Public API Documentation
Authentic Jobs is a job board website, which provides a public API for developers to search and receive job listings information. In this article, we will go through the API documentation and provide JavaScript example code for each endpoint.
Requirements
To use the Authentic Jobs public API, an API key is required. You can obtain a key by signing up for the Authentic Jobs developer program.
Endpoints
The Authentic Jobs public API provides several endpoints for developers to use. Here is a list of all currently available endpoints:
GET /api/posts/search
This endpoint allows you to search for job listings based on keywords, location, and category.
Example code:
const apiKey = 'your_api_key';
const url = `https://authenticjobs.com/api/posts/search/?api_key=${apiKey}&keywords=javascript&category=1`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
GET /api/categories
This endpoint returns a list of all job categories available on Authentic Jobs.
Example code:
const apiKey = 'your_api_key';
const url = `https://authenticjobs.com/api/categories/?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
GET /api/tags
This endpoint returns a list of all job tags available on Authentic Jobs.
Example code:
const apiKey = 'your_api_key';
const url = `https://authenticjobs.com/api/tags/?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
GET /api/posts/:id
This endpoint returns information about a specific job listing.
Example code:
const apiKey = 'your_api_key';
const postId = 123;
const url = `https://authenticjobs.com/api/posts/${postId}/?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
GET /api/posts/recent
This endpoint returns a list of the most recent job listings on Authentic Jobs.
Example code:
const apiKey = 'your_api_key';
const url = `https://authenticjobs.com/api/posts/recent/?api_key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
Conclusion
The Authentic Jobs public API provides developers with a powerful tool to search and retrieve job listings information. This article has provided JavaScript example code for each available endpoint. With this information, you can integrate Authentic Jobs job listings data into your own websites or applications.