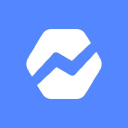
Baremetrics
FinanceExploring the Baremetrics API Documentation
As a developer, working with public APIs is an essential part of your job. One such API that you might find useful is the Baremetrics API. The API provides data related to your company's metrics, including your customer data, revenue, MRR, and more.
The API is available as a REST API, and you can use the axios
package in JavaScript to make API calls. In this blog post, we'll explore how to use the Baremetrics API by looking at some example code in JavaScript.
Getting Started
Before you begin using the Baremetrics API, you need to generate your API key. You can do this by creating an account on Baremetrics and generating a new API key from the dashboard. Once you have the API key, you can start making API calls using JavaScript.
We'll be using the axios
package to make API calls. So, before we start, make sure you have installed axios
using npm.
npm install axios
Example Code
In the following sections, we'll look at some example code that demonstrates how to use the Baremetrics API in JavaScript. Each code snippet uses a specific API endpoint and shows how to make a request and handle the response.
Customers API
The Customers API provides customer data for your company. You can get a list of customers, add new customers, update customer data, and more.
const axios = require('axios');
const API_KEY = 'your-api-key';
const API_ENDPOINT = 'https://api.baremetrics.com/v1';
const headers = {
Authorization: `Bearer ${API_KEY}`,
Accept: 'application/json',
'Content-Type': 'application/json',
};
// Get a list of all customers
axios.get(`${API_ENDPOINT}/customers`, { headers })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
// Add a new customer
const customerData = {
name: 'John Doe',
email: 'johndoe@example.com',
plan: 'starter',
};
axios.post(`${API_ENDPOINT}/customers`, customerData, { headers })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Metrics API
The Metrics API provides your company's financial data, such as revenue, MRR, and other metrics.
// Get all metrics for the last 30 days
axios.get(`${API_ENDPOINT}/metrics`, { headers, params: { period: '30_days' } })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
// Get MRR for the current month
axios.get(`${API_ENDPOINT}/metrics/mrr`, { headers })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
Subscriptions API
The Subscriptions API provides data related to your company's subscriptions. You can get a list of subscriptions, add new subscriptions, cancel subscriptions, and more.
// Get a list of all subscriptions
axios.get(`${API_ENDPOINT}/subscriptions`, { headers })
.then((response) => {
console.log(response.data);
})
.catch((error) => {
console.error(error);
});
// Cancel a subscription
axios.delete(`${API_ENDPOINT}/subscriptions/:id`, { headers })
.then(() => {
console.log('Subscription canceled');
})
.catch((error) => {
console.error(error);
});
Conclusion
In this blog post, we explored how to use the Baremetrics API by looking at some example code in JavaScript. We covered the Customer API, Metrics API, and Subscriptions API. By using these APIs, you can get valuable data related to your company's metrics, which can help you make informed decisions. Happy coding!