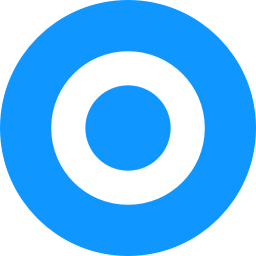
Base64.ai
Machine LearningDecode and Encode Images with Base64.ai Public API
Base64 encoding is a way of converting images into strings of text that can be easily transferred between different platforms. This can be used to optimize the user experience, as images can be loaded faster and more efficiently. The Base64.ai Public API provides a simple way to encode and decode images using different programming languages, including JavaScript.
To use the Base64.ai API with JavaScript, you need to follow these steps:
- Sign up for a free account on the Base64.ai website.
- Obtain your API key, which will be used to authenticate your API calls.
- Choose the API endpoint you want to use. Base64.ai offers different APIs for different image-related tasks, such as image compression, image optimization, image resizing, and more.
- Set up your API call with JavaScript. This involves formatting the request in JSON and sending it to the Base64.ai server.
- Receive the API response, which will include the encoded or decoded image.
Here are some example code snippets that show how to use the Base64.ai Public API with JavaScript:
Encode an Image to Base64
var imageUrl = 'https://example.com/image.jpg';
var apiKey = 'YOUR_API_KEY';
var requestData = {
'image_url': imageUrl,
'api_key': apiKey
};
var request = new XMLHttpRequest();
request.open('POST', 'https://api.base64.ai/v1/encode');
request.setRequestHeader('Content-Type', 'application/json');
request.onload = function() {
if (request.status === 200) {
var response = JSON.parse(request.responseText);
var encodedImage = response['data']['image_base64'];
console.log(encodedImage);
} else {
console.log('Error occurred while encoding image.');
}
};
request.send(JSON.stringify(requestData));
Decode an Image from Base64
var encodedImage = 'BASE64_ENCODED_IMAGE';
var apiKey = 'YOUR_API_KEY';
var requestData = {
'image_base64': encodedImage,
'api_key': apiKey
};
var request = new XMLHttpRequest();
request.open('POST', 'https://api.base64.ai/v1/decode');
request.setRequestHeader('Content-Type', 'application/json');
request.onload = function() {
if (request.status === 200) {
var response = JSON.parse(request.responseText);
var decodedImage = response['data']['image_url'];
console.log(decodedImage);
} else {
console.log('Error occurred while decoding image.');
}
};
request.send(JSON.stringify(requestData));
These code snippets demonstrate how to use the Base64.ai Public API with JavaScript to encode and decode images. With this simple approach, you can optimize your website by loading images faster and more efficiently. Give it a try today!