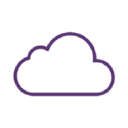
Bible API
Open DataUsing the bibleapi.co API Docs
The bibleapi.co is a public API that provides access to the Bible in different translations and versions. This API is great for building Bible-related applications or tools that require accessing specific Bible verses, chapters, or books.
In this post, we will explore how to use the bibleapi.co API and provide some example code snippets in JavaScript.
Getting Started
Before we begin, make sure you have signed up for an API key in order to use the bibleapi.co API. Once you have your API key, you are ready to get started.
Making API Requests
To interact with the BibleAPI, you will need to make an HTTP request to the API endpoint. The base URL for this endpoint is https://bibleapi.co/api
.
API Example Code
Retrieving a Verse
To retrieve a verse, you will need to make a GET request to the /verses
endpoint with the chapterId
and verseNumber
parameters. Here's an example code snippet in JavaScript:
const apiKey = 'YOUR_API_KEY';
const chapterId = 'jhn.1';
const verseNumber = '1';
fetch(`https://bibleapi.co/api/verses/${chapterId}/${verseNumber}?version=asv`, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
In the example above, we are using the fetch()
function to make a GET request to the /verses
endpoint with the chapterId
and verseNumber
parameters. We also include our API key in the Authorization
header.
Retrieving a Chapter
To retrieve a chapter, you will need to make a GET request to the /chapters
endpoint with the chapterId
parameter. Here's an example code snippet in JavaScript:
const apiKey = 'YOUR_API_KEY';
const chapterId = 'jhn.1';
fetch(`https://bibleapi.co/api/chapters/${chapterId}?version=asv`, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
In the example above, we are using the fetch()
function to make a GET request to the /chapters
endpoint with the chapterId
parameter. We also include our API key in the Authorization
header.
Retrieving a Book
To retrieve a book, you will need to make a GET request to the /books
endpoint with the bookId
parameter. Here's an example code snippet in JavaScript:
const apiKey = 'YOUR_API_KEY';
const bookId = 'john';
fetch(`https://bibleapi.co/api/books/${bookId}?version=asv`, {
headers: {
'Authorization': `Bearer ${apiKey}`
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
In the example above, we are using the fetch()
function to make a GET request to the /books
endpoint with the bookId
parameter. We also include our API key in the Authorization
header.
Conclusion
The bibleapi.co API is a great resource for developers who are building Bible-related applications or tools. With the code snippets provided above, you now know how to use the API to retrieve verses, chapters, and books. Happy coding!