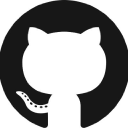
Breaking Bad Quotes
VideoUsing The Breaking Bad Quotes Public API
If you're a fan of the show Breaking Bad, you'll be excited to learn that there is a public API available that you can use to retrieve and display quotes from the show. In this blog, we'll learn how to use the Breaking Bad Quotes API with JavaScript.
Getting Started
To get started, we need to understand the structure of the API. The Breaking Bad Quotes API is a RESTful API that returns quotes from the show as JSON objects. The API is located at https://breaking-bad-quotes.herokuapp.com/v1/quotes.
API Example Code
Here is some code that you can use to retrieve quotes from the API using JavaScript:
fetch('https://breaking-bad-quotes.herokuapp.com/v1/quotes')
.then(response => response.json())
.then(data => {
// Do something with the data
console.log(data[0].quote);
});
This code uses the fetch
function to send a GET request to the API endpoint. Once the response is received, it is parsed as JSON and the data is available for use in the callback function. In this example, we simply access the quote property of the first element in the returned array and log it to the console.
Here's another example that demonstrates how to use the API with the XMLHttpRequest
object:
let xhr = new XMLHttpRequest();
xhr.open('GET', 'https://breaking-bad-quotes.herokuapp.com/v1/quotes');
xhr.responseType = 'json';
xhr.onload = function() {
// Do something with the data
console.log(xhr.response[0].quote);
};
xhr.send();
Here, we create an XMLHttpRequest
object and send a GET request to the API endpoint. Once the response is received, it is parsed as JSON and the data is available for use in the onload
callback function. Again, we simply access the quote property of the first element in the returned array and log it to the console.
Conclusion
In this blog, we've learned how to use the Breaking Bad Quotes API with JavaScript. By sending a GET request to the API endpoint, we can retrieve and display quotes from the show. So, if you're a fan of Breaking Bad, be sure to check out this API and start using it in your projects!