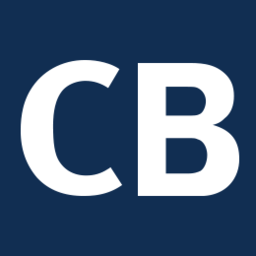
Census.gov
GovernmentExploring the U.S. Census Bureau's Public API Docs
The U.S. Census Bureau offers a public API that provides access to various data sets, surveys, and programs. With this API, developers and data analysts can retrieve data, analyze trends, and create customized visualizations. In this blog post, we'll explore how to use this public API and provide some example JavaScript code.
Prerequisites
Before we start exploring the U.S. Census Bureau's public API, we need to make sure we have all the necessary tools installed on our system. Here are the tools and libraries you'll need:
- Node.js: We'll use Node.js to execute our JavaScript code.
- Axios: Axios is a popular library for making HTTP requests from Node.js.
- dotenv: dotenv allows us to store sensitive API keys in an environment file.
Once you have these tools installed, let's start exploring the U.S. Census Bureau's public API.
Getting Started
First, we need to obtain an API key from the U.S. Census Bureau's website. Head over to their website and sign up for a key.
Once you have your API key, create a .env
file in the root directory of your project and add your key as follows:
API_KEY=your-api-key-goes-here
Now, let's install the required libraries by running the following commands in your terminal:
npm install axios dotenv
With the setup complete, we can now start querying the U.S. Census Bureau's public API.
Example Code
Fetching Population Data by State
Let's start by fetching population data by state. The getPopulationData
function makes a GET request to the Census Bureau API endpoint /data/2019/pep/population
. We pass getPopulationData
a state FIPS code and the API key as query parameters.
const axios = require('axios');
require('dotenv').config();
const API_KEY = process.env.API_KEY;
async function getPopulationData(stateFips) {
const url = `https://api.census.gov/data/2019/pep/population?get=POP&for=state:${stateFips}&key=${API_KEY}`;
try {
const response = await axios.get(url);
const [headers, data] = response.data;
const [state, population] = data;
console.log(`Population of ${state}: ${population}`);
} catch (error) {
console.error(error);
}
}
getPopulationData(6); // California
getPopulationData(48); // Texas
Fetching Data by Zip Code
Next, let's fetch data by zip code. This time we'll use the /data/2019/pep/population
endpoint to retrieve population data by 5-digit zip code.
const axios = require('axios');
require('dotenv').config();
const API_KEY = process.env.API_KEY;
async function getPopulationDataByZip(zipCode) {
const url = `https://api.census.gov/data/2019/pep/population?get=POP&for=zip%20code%20tabulation%20area:${zipCode}&key=${API_KEY}`;
try {
const response = await axios.get(url);
const [headers, data] = response.data;
const [zip, population] = data;
console.log(`Population of ${zip}: ${population}`);
} catch (error) {
console.error(error);
}
}
getPopulationDataByZip(90210); // Beverly Hills, CA
getPopulationDataByZip(10001); // New York, NY
Fetching Data by County and Year
Lastly, let's fetch data by county and year. This time we'll use the /data/2019/acs/acs5
endpoint to retrieve total population and median household income by county and year.
const axios = require('axios');
require('dotenv').config();
const API_KEY = process.env.API_KEY;
async function getCountyData(county, state, year) {
const url = `https://api.census.gov/data/2019/acs/acs5?get=B01003_001E,B19013_001E&for=county:${county}&in=state:${state}&year=${year}&key=${API_KEY}`;
try {
const response = await axios.get(url);
const [headers, data] = response.data;
const [population, income] = data[0];
console.log(`${county}, ${state}: population - ${population} | median income - ${income}`);
} catch (error) {
console.error(error);
}
}
getCountyData('001', '06', '2019'); // Alameda County, CA
getCountyData('081', '48', '2019'); // Harris County, TX
Conclusion
The U.S. Census Bureau's public API provides access to a wealth of data that can be used for a variety of purposes. In this blog post, we've explored how to use this API and provided some example JavaScript code. With this knowledge, you can now start experimenting with different data sets, queries, and visualizations. Happy exploring!