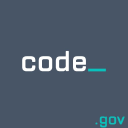
Code.gov
GovernmentIntroduction to code.gov API documentation
Code.gov is an online platform that provides access to the federal government's open-source software projects. If you want to access the code.gov data programmatically, you can use the API provided by code.gov. In this blog post, we will explore how to use the code.gov API in JavaScript, with code examples.
Getting Started with the code.gov API
Before you start using the code.gov API, you need to register for an API key. You can do this by visiting the API key registration page on the code.gov website. Once you have registered, you will receive an API key that you can use to access the code.gov API.
Example code in JavaScript
Below are some examples of how to use the code.gov API in JavaScript:
Example 1: Fetching repository details
const API_KEY = 'YOUR_API_KEY';
const REPO_API_URL = 'https://api.code.gov/repos';
let options = {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Api-Key': API_KEY
}
};
fetch(REPO_API_URL, options)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This example code fetches repository details from the code.gov API using the fetch
function in JavaScript. You need to provide your API key in the headers
object. The console.log(data)
statement prints the fetched data to the console.
Example 2: Searching for a repository
const API_KEY = 'YOUR_API_KEY';
const REPO_API_URL = 'https://api.code.gov/repos';
const SEARCH_TERM = 'example';
let options = {
method: 'GET',
headers: {
'Content-Type': 'application/json',
'Api-Key': API_KEY
}
};
fetch(`${REPO_API_URL}?q=${SEARCH_TERM}`, options)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This example code searches for repositories containing the search term "example" using the fetch
function in JavaScript. You need to provide your API key in the headers
object. The console.log(data)
statement prints the search results to the console.
Conclusion
In this blog post, we have explored how to use the code.gov API in JavaScript, with code examples. With these examples, you can start exploring the vast open-source software projects made available to you by the U.S. federal government!