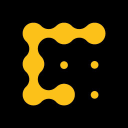
CoinDesk
FinanceUsing the CoinDesk API
If you're interested in using cryptocurrency information in your applications, the CoinDesk API provides a way to access real-time and historical data on Bitcoin and other cryptocurrencies. In this post, we'll explore how to use the CoinDesk API with JavaScript, leveraging examples provided by the official documentation.
Getting Started
Before we begin, we need to obtain an API key. You can obtain a free API key by signing up on the CoinDesk API website. Once you have your API key, we're ready to start accessing the data.
We'll be using JavaScript for our examples, and since most modern JavaScript is running in the browser, we'll be using the fetch()
method to interact with the API. Here's an example of how to access the current Bitcoin price in USD:
fetch('https://api.coindesk.com/v1/bpi/currentprice/USD.json')
.then(response => response.json())
.then(data => {
console.log(`Current Bitcoin Price: ${data.bpi.USD.rate}`);
})
.catch(error => {
console.error("Error fetching current Bitcoin price:", error);
});
This code sends a GET
request to the CoinDesk API, asking for the current Bitcoin price in USD. The response is in JSON format, which we access by calling the .json()
method on the response.
The resulting data
object contains a few different keys, but the bpi
key contains the actual price data. We extract it by using .
notation to access the USD.rate
key.
Historical Data
In addition to the current Bitcoin price, the CoinDesk API provides access to historical pricing data. Here's an example of how to retrieve the Bitcoin price from exactly one year ago:
const oneYearAgo = new Date(Date.now() - (365 * 24 * 60 * 60 * 1000));
const url = `https://api.coindesk.com/v1/bpi/historical/close.json?start=${oneYearAgo.toISOString().slice(0,10)}&end=${new Date().toISOString().slice(0,10)}`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(`Price one year ago: ${data.bpi[Object.keys(data.bpi)[0]]}`);
})
.catch(error => {
console.error("Error fetching historical Bitcoin price:", error);
});
This code sets up a Date
object for exactly one year ago, constructs a string URL for the API request, and sends it via fetch()
. This time, the API is returning a JSON object containing pricing data for each day during the specified range. We extract the first value from the bpi
object (which represents the oldest data point) and output it to the console.
Conclusion
The CoinDesk API provides a wealth of information on Bitcoin and other cryptocurrencies, and with the examples provided above, you should be well-equipped to start integrating this data into your JavaScript projects. Stay curious, and happy coding!