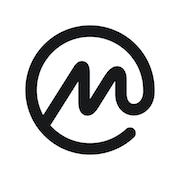
CoinMarketCap
FinanceExploring the Coinmarketcap Public API
Coinmarketcap is one of the most popular websites for tracking cryptocurrency prices. They offer a free public API to access their cryptocurrency data. In this article, we'll explore how to use this API and provide code examples in JavaScript.
Prerequisites
Before we start, make sure that you have Node.js installed on your computer. You can download it from the official website.
Getting Started
First, we need to create an account on Coinmarketcap to obtain an API Key. Once you've created an account, go to your account dashboard and click on the "API" tab. From here, you can generate your API Key.
Using the Coinmarketcap Public API
The Coinmarketcap Public API supports both HTTP and HTTPS requests. The base URL for the API is https://pro-api.coinmarketcap.com/v1/
.
To make requests to the API, we need to include our API Key as a header with each request. Here's an example using the Fetch API:
const fetch = require("node-fetch");
const apiKey = "YOUR_API_KEY";
fetch("https://pro-api.coinmarketcap.com/v1/cryptocurrency/map", {
headers: {
"X-CMC_PRO_API_KEY": apiKey
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
This code sends a GET request to the cryptocurrency/map
endpoint of the Coinmarketcap API and logs the response data to the console.
Example API Requests
Here are some example requests that you can make to the Coinmarketcap Public API:
List of Cryptocurrencies
fetch("https://pro-api.coinmarketcap.com/v1/cryptocurrency/listings/latest?limit=10", {
headers: {
"X-CMC_PRO_API_KEY": apiKey
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
This code sends a GET request to the cryptocurrency/listings/latest
endpoint with a limit of 10 and logs the response data to the console.
Price Conversion
fetch("https://pro-api.coinmarketcap.com/v1/tools/price-conversion?amount=1&symbol=BTC&convert=USD", {
headers: {
"X-CMC_PRO_API_KEY": apiKey
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
This code sends a GET request to the tools/price-conversion
endpoint to convert 1 Bitcoin to US dollars and logs the response data to the console.
Historical Data
fetch("https://pro-api.coinmarketcap.com/v1/cryptocurrency/quotes/historical?symbol=BTC&time_start=2021-09-01T00%3A00%3A00.000Z&time_end=2021-09-30T00%3A00%3A00.000Z", {
headers: {
"X-CMC_PRO_API_KEY": apiKey
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(err => console.error(err));
This code sends a GET request to the cryptocurrency/quotes/historical
endpoint to get historical data for Bitcoin from September 1 to September 30, 2021 and logs the response data to the console.
Conclusion
The Coinmarketcap Public API provides a powerful way to access cryptocurrency data. With the examples we provided in this article, you now have a solid starting point for using the API.