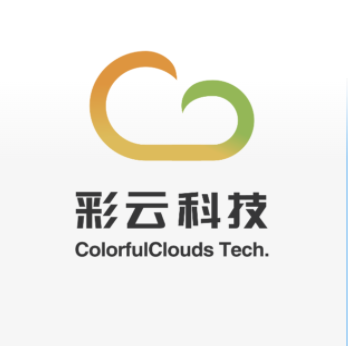
Colorful Clouds Weather API
WeatherExploring the ColorfulClouds Weather API
The ColorfulClouds Weather API is a public API that allows developers to access information about weather conditions in different locations. This API provides various endpoints that return current and forecast weather conditions for a specific location.
In this blog, we will explore how to use this API through JavaScript and provide several example codes.
Getting Started
Before we dive into coding, we need to get an API key. We can obtain this key from the ColorfulClouds website. Once we have our key, we can use it to call the API endpoints.
Here is the basic structure of an API request:
const key = "YOUR-API-KEY";
const url = `https://api.caiyunapp.com/v2.5/${key}/XXX/YYY`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error(error));
In the code above, we declare our API key, set the URL for accessing the endpoint, and make a fetch request. We then parse the JSON data received from the endpoint and log it to the console.
Example Codes
Get Current Weather Conditions
Here is an example code that shows the current weather conditions for a location:
const key = "YOUR-API-KEY";
const url = `https://api.caiyunapp.com/v2.5/${key}/121.6544,25.1552/realtime.json`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data.result.temperature);
})
.catch(error => console.error(error));
In the code above, we use the realtime.json
endpoint to retrieve the current weather conditions for the specified location. We then log the temperature to the console.
Get Daily Weather Forecast
Here is an example code that shows the daily weather forecast for a location:
const key = "YOUR-API-KEY";
const url = `https://api.caiyunapp.com/v2.5/${key}/121.6544,25.1552/daily.json`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data.result.daily.temperature[0]);
})
.catch(error => console.error(error));
In the code above, we use the daily.json
endpoint to retrieve the daily weather forecast for the specified location. We then log the temperature for the first day to the console.
Get Hourly Weather Forecast
Here is an example code that shows the hourly weather forecast for a location:
const key = "YOUR-API-KEY";
const url = `https://api.caiyunapp.com/v2.5/${key}/121.6544,25.1552/hourly.json`;
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data.result.hourly.temperature[0]);
})
.catch(error => console.error(error));
In the code above, we use the hourly.json
endpoint to retrieve the hourly weather forecast for the specified location. We then log the temperature for the first hour to the console.
Conclusion
In this blog, we explored the ColorfulClouds Weather API and provided example codes to retrieve the current weather conditions, daily weather forecast, and hourly weather forecast for a specific location. With these codes, we can easily integrate this API into our web or mobile applications.