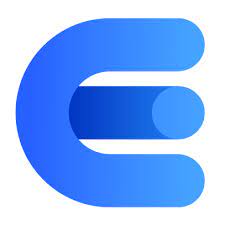
Currencyapi
Currency ExchangeIntroduction to CurrencyAPI
CurrencyAPI is a public API that provides real-time exchange rates for currencies from around the world. The API offers data from over 170 currencies and is frequently updated, ensuring that the information is accurate and up-to-date.
In this blog, we will explore how to use CurrencyAPI and provide sample code in JavaScript.
Getting Started
Before we start using the CurrencyAPI, we need to register for an API key, which we will use to authenticate our requests. The registration is free and just takes a few minutes.
Once you have registered, you can use the following endpoint to access the latest exchange rates:
https://currencyapi.com/api/v1/rates?key=YOUR_API_KEY
To get information about a specific currency, use the following endpoint:
https://currencyapi.com/api/v1/rates?base=USD&key=YOUR_API_KEY
Sample Code
The following code examples show how to retrieve exchange rates and currency information using CurrencyAPI.
Retrieving Exchange Rates
To retrieve the latest exchange rates, we can use the fetch() function in JavaScript. Here's an example code snippet:
fetch('https://currencyapi.com/api/v1/rates?key=YOUR_API_KEY')
.then(response => response.json())
.then(data => {
console.log(data); // prints the latest exchange rates
})
.catch(error => {
console.error(error);
});
Retrieving Information About a Specific Currency
To retrieve information about a specific currency, we can modify the previous code snippet to include the currency code in the URL. Here's an example code snippet:
fetch('https://currencyapi.com/api/v1/rates?base=USD&key=YOUR_API_KEY')
.then(response => response.json())
.then(data => {
console.log(data); // prints information about USD
})
.catch(error => {
console.error(error);
});
Conclusion
CurrencyAPI is a powerful and straightforward API that provides real-time exchange rates and currency information. With sample code in JavaScript, you can easily integrate this API into your web application. Try it out for yourself and see how easy it is to work with CurrencyAPI!