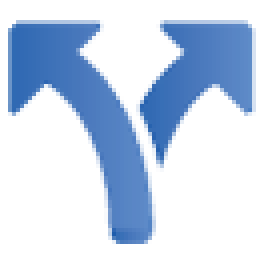
Currencylayer
Currency ExchangeCurrency Layer API Documentation
Currencylayer provides a comprehensive API for real-time and historical exchange rates, including a secure and reliable service with 256-bit SSL encryption and backed by 24/7 support.
In this blog post, we will explore the documentation for Currency Layer API and provide some example code in JavaScript.
Getting started
Before you can use the Currency Layer API, you need to sign up for a free account and get an API access key. The access key is required for all requests to the API.
Once you have obtained your access key, you can start making requests to the API. The API supports both HTTP and HTTPS protocols.
API Requests
The Currency Layer API supports a wide range of requests, including exchange rate quotes, historical rates, time-series data, currency conversion, and more.
Here is a sample request for getting the latest exchange rate:
const access_key = 'API_KEY';
const url = `http://apilayer.net/api/live?access_key=${access_key}`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data));
This example uses the fetch
API to make an HTTP request to the API endpoint and log the response.
Response Format
The response format for all requests to the Currency Layer API is JSON.
Here is an example response for the latest exchange rate request:
{
"success": true,
"terms": "https://currencylayer.com/terms",
"privacy": "https://currencylayer.com/privacy",
"timestamp": 1614325980,
"source": "USD",
"quotes": {
"USDAED": 3.673205,
"USDAFN": 77.385787,
"USDALL": 102.64999,
...
}
}
The response JSON object includes the following keys:
success
: A boolean value indicating whether the request was successfulterms
: A URL for the terms of service for the Currency Layer APIprivacy
: A URL for the privacy policy for the Currency Layer APItimestamp
: A Unix timestamp representing the time when the exchange rates were collectedsource
: The source currency for the exchange ratesquotes
: A JSON object containing the exchange rates for each currency
Error Handling
The Currency Layer API returns error messages as JSON strings when there is an issue with a request.
Here is an example error response:
{
"success": false,
"error": {
"code": 101,
"type": "missing_access_key",
"info": "You have not supplied an API Access Key. [Required format: access_key=YOUR_ACCESS_KEY]"
}
}
The error JSON object includes the following keys:
success
: A boolean value indicating whether the request was successfulerror
: A JSON object containing the error message, which includes the following keys:code
: An integer representing the error codetype
: A string representing the error typeinfo
: A string representing additional information about the error
Conclusion
The Currency Layer API provides a robust and feature-rich solution for obtaining real-time and historical exchange rates. With a simple and easy-to-use API and extensive documentation, it is a great choice for developers looking to build currency conversion, financial, or international applications.
We hope this blog post has provided you with a useful introduction to the Currency Layer API and some example code in JavaScript to get you started. Happy coding!