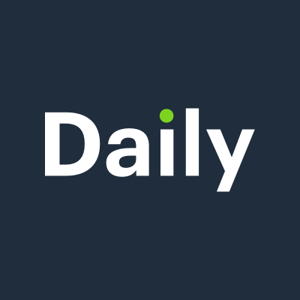
Daily.co
VideoA Beginner's Guide to Daily.co's Public API Docs
Daily.co's public API is an accessible and easy-to-use tool for developers who want to embed video chat and conferencing capabilities into their apps and websites. In this guide, we will walk you through the basic concepts and functionalities of the API and provide you with sample code in JavaScript to help you get started.
Getting Started
Before you can use the Daily.co API, you need to sign up for a free account. Once you've done that, you can use your API key to authenticate your app or website with the Daily.co servers.
Basic Concepts
The Daily.co API works on the concept of rooms and users. A room is where a video chat or conferencing session takes place, and a user is someone who participates in that session. When you create a room, the API generates a unique URL that you can use to invite other users to join the room.
API Endpoints
The Daily.co API provides a number of endpoints that you can use to control the behavior of your video chat or conferencing session. Here are a few of the most common endpoints and their functions:
-
/call
: This endpoint is used to initiate a video chat or conferencing session. To use this endpoint, you need to provide the name of the room you want to create, as well as any options you want to set for the session. -
/room/{name}
: This endpoint is used to get information about a specific room, such as the number of users currently connected to the room. -
/room/{name}/users
: This endpoint is used to get a list of users currently connected to a specific room. -
/room/{name}/update
: This endpoint is used to update the properties of a specific room, such as the name of the room or the maximum number of users allowed in the room.
Sample Code
Here is an example of how you can use the Daily.co API to create a room and invite other users to join the session:
const daily = require('@daily-co/daily-js');
// Replace YOUR_API_KEY with your actual API key
daily.setApiKey('YOUR_API_KEY');
// This function creates a new room and returns the URL for that room
async function createRoom(roomName) {
const callOptions = {
maxParticipants: 10,
};
const callObject = await daily.createCallObject(callOptions);
const createdCall = await daily.createCall(callObject);
// Update the room name to match the provided name
await createdCall.update({ properties: { name: roomName } });
return createdCall.url;
}
// This function invites a user to join the specified room
async function inviteUser(roomUrl, userEmail) {
const callObject = await daily.joinCall({ url: roomUrl });
const joinOptions = {
userName: userEmail,
};
const userObject = await daily.createUserObject(joinOptions);
const userToken = await daily.createUserToken(callObject.url, userObject);
return userToken;
}
// Example usage
async function main() {
const roomUrl = await createRoom('My Room');
console.log(`Room URL: ${roomUrl}`);
const user1Token = await inviteUser(roomUrl, 'user1@example.com');
const user2Token = await inviteUser(roomUrl, 'user2@example.com');
console.log(`User 1 Token: ${user1Token}`);
console.log(`User 2 Token: ${user2Token}`);
}
main();
In this example, we used the createCallObject
and createCall
functions to create a new room, and the joinCall
, createUserObject
, and createUserToken
functions to invite users to the room and obtain tokens for their authentication. If you want to know more about the full range of functionalities of the Daily.co API, be sure to check out their public API documentation.
Conclusion
We hope that this beginner's guide has helped you understand the basics of the Daily.co API and how you can use it in your own projects. Whether you're building a video chat or conferencing app from scratch or adding video capabilities to an existing app, the Daily.co API is a useful and powerful tool that you can use to get the job done with ease.