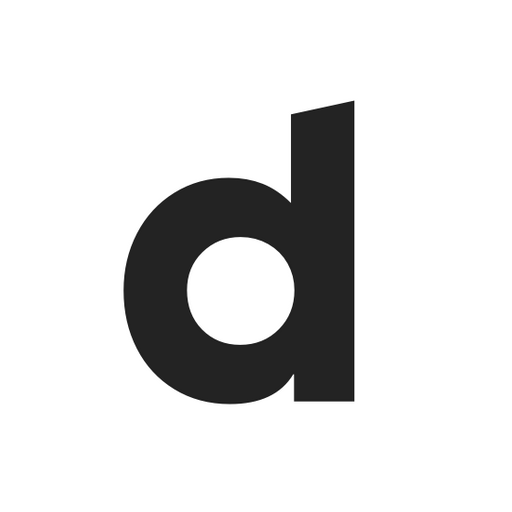
Dailymotion
VideoExploring the DailyMotion API with JavaScript
DailyMotion is a popular video-sharing website that allows users to watch and share videos. The site also provides an API that developers can use to access video-related data and functionalities. In this article, we will explore the DailyMotion API, discuss its features, and provide examples of API calls using JavaScript.
Getting Started
To get started with the DailyMotion API, you need to create an account and register your application. Once registered, you will receive an API key that you can use to access the API. The API supports both OAuth2 and API key authentication methods. In this article, we will be using the API key authentication method.
API Endpoints and Features
The DailyMotion API exposes several endpoints for video-related functionalities, such as retrieving video information, searching for videos, and uploading videos. Here are some of the key endpoints and features provided by the API:
GET /videos
: Retrieve information about a video.GET /videos/:id/comments
: Retrieve comments for a video.POST /videos
: Upload a video.GET /videos/search
: Search for videos based on a query.
Performing API calls with JavaScript
To perform API calls with JavaScript, we can use the fetch
function provided by the browser. Here's an example of how to retrieve information about a video:
const videoId = 'x7yr2sm';
const apiKey = 'YOUR_API_KEY';
fetch(`https://api.dailymotion.com/video/${videoId}?fields=title,url,description&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are making a GET
request to retrieve information about a video with ID x7yr2sm
. We are also specifying the fields title
, url
, and description
that we want to retrieve. Finally, we include the API key as a query parameter.
Here's an example of how to search for videos:
const searchQuery = 'surfing';
const apiKey = 'YOUR_API_KEY';
fetch(`https://api.dailymotion.com/videos?search=${searchQuery}&fields=title,url,thumbnail_240_url&apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
In this example, we are making a GET
request to search for videos that match the query surfing
. We are also specifying the fields title
, url
, and thumbnail_240_url
that we want to retrieve. Finally, we include the API key as a query parameter.
Conclusion
In this article, we explored the DailyMotion API and its features. We also provided examples of API calls using JavaScript. By using the DailyMotion API, developers can build applications that integrate with the video-sharing website and provide additional functionalities to users.