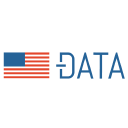
Data.gov
GovernmentA Beginner's Guide to Using the Public API Docs on api.data.gov
If you are looking for publicly available data from the US government, you might want to check out api.data.gov. This website provides a vast collection of APIs that you can use to access various datasets. In this guide, we will show you how to get started using the Public API Docs on api.data.gov using JavaScript.
Step 1: Register for an API Key
Before you can start using any of the APIs available on api.data.gov, you need to register and obtain an API key. The API key is a unique identifier that allows the website to track who is using its services. To register, follow these steps:
- Go to the Registration Page for api.data.gov.
- Fill out the registration form, which includes basic information, like your name, email address, and postal code.
- Once you submit the form, you will receive an email confirmation that your registration is completed.
- Log in to api.data.gov using your new account information and navigate to the "My Account" section to get your API key.
Step 2: Choose an API
Once you have obtained an API key, you can use it to access any of the APIs available on api.data.gov. You can browse through the API Catalog to find an API that meets your needs. For this guide, we will use the USDA National Nutrient Database API. This API provides information on the nutritional content of various food items.
Step 3: Test the API
Before you can start writing code, you need to test the API to make sure that it works as expected. The easiest way to do this is to use the API's web test console, which is available on its documentation page. For example, to test the USDA National Nutrient Database API, you can go to its documentation page and click on the "try it out" button under the "List Search" section.
Once you click the button, the console will load, and you can enter the parameters for your API call. For example, to search for food items that contain the word "banana," you can set the "q" parameter to "banana" and click "send." The console will return a response in JSON format that contains information on the food items that match your search criteria.
Step 4: Write Your JavaScript Code
Now that you have tested the API, you can start writing your JavaScript code to access it. Here is some sample code that demonstrates how to use the USDA National Nutrient Database API to search for food items that contain the word "banana:"
const apiKey = "YOUR_API_KEY";
const query = "banana";
const apiUrl = `https://api.nal.usda.gov/ndb/search/?format=json&q=${query}&api_key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => console.log(data));
In this code, we first define our API key and search query. We then construct the API URL by combining the base URL, our search query, and our API key. We use the fetch
function to make a GET request to the API URL and parse the JSON response using response.json()
. Finally, we log the parsed data to the console.
Conclusion
Using the Public API Docs on api.data.gov can be a great way to access publicly available data from the US government. By registering for an API key, choosing an API, testing the API, and writing your JavaScript code, you can easily start accessing the available datasets and using them in your web applications. Happy coding!