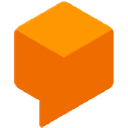
Dialogflow
Machine LearningExploring the Dialogflow API with JavaScript
If you are looking to build conversational interfaces or chat bots powered by artificial intelligence, Dialogflow is one tool you should consider. With Dialogflow's powerful Natural Language Understanding (NLU) engine, you can quickly train your bots to recognize user intents and entities, and respond accordingly.
In this blog post, we will explore how to use the Dialogflow API with JavaScript, and provide you with some sample code you can use to get started.
Setting up Dialogflow
Before you can start using the Dialogflow API, you need to set up a Dialogflow agent. Agents are entities in Dialogflow that you create to handle conversations with your users. You can create an agent by going to the Dialogflow console (https://console.dialogflow.com), logging in with your Google account, and clicking on the "Create Agent" button.
Once you have created your agent, you can create intents that define the various things your bot can do. You can also define entities, which are specific pieces of information your bot needs to understand (like dates, times, places, or names).
Connecting to the Dialogflow API
Once you have set up your Dialogflow agent, you can use the Dialogflow API to interact with it programmatically. To use the API, you will need to obtain an API key. You can create an API key in the Dialogflow console by going to the "Settings" tab, clicking on the "API keys" link, and clicking on the "Create new key" button.
With your API key in hand, you can use any HTTP client library to interact with the Dialogflow API. In this post, we will use the popular Axios library for making HTTP requests in JavaScript.
const axios = require('axios');
const baseURL = 'https://api.dialogflow.com/v1';
const apiKey = '<YOUR-API-KEY>';
const sessionId = '<YOUR-SESSION-ID>';
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json; charset=utf-8'
};
axios.post(`${baseURL}/query?v=20150910`, {
query: 'Hello',
lang: 'en',
sessionId: sessionId
}, { headers: headers })
.then(response => {
console.log(response.data.result.fulfillment.speech);
})
.catch(error => {
console.log(error);
});
In this code, we are using the axios.post()
method to send a POST request to the Dialogflow API's /query
endpoint. We are passing in an object with three properties:
query
: The user's query or messagelang
: The language the query is in (we're using English in this case)sessionId
: A unique ID that identifies the conversation session with the user
We're also passing in an object with headers that include our API key as an Authorization
header.
When the API responds, we're logging the response's result.fulfillment.speech
property to the console. This is the text that the bot will respond with.
Conclusion
In this post, we showed you how to use the Dialogflow API with JavaScript to build chat bots and conversational interfaces. With the power of NLU, you can quickly train your bots to recognize user intents and entities, and respond with appropriate actions.
We hope this code snippet was helpful. To learn more about the Dialogflow API, check out the official documentation at https://cloud.google.com/dialogflow/docs/apis.