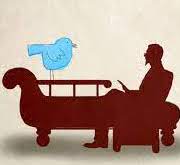
ElonMush
NewsElon Mush API Documentation
Elon Mush is a public API that provides access to SpaceX launch data, news, and more. In this guide, we'll cover everything you need to know to get started with the Elon Mush API, including API endpoints, authentication, and JavaScript examples.
Getting Started
To use the Elon Mush API, you'll need to sign up for an API key. This will allow you to access the various endpoints that are available. To get started, go to the API documentation page and click on the "Get API Key" button. This will generate an API key for you that you can use to access the API.
API Endpoints
Once you have your API key, you can use it to access the various endpoints available in the Elon Mush API. Here are some of the most commonly used endpoints:
Get Latest Launch
https://api.elonmu.sh/v1/launches/latest?apikey=YOUR_API_KEY
Get Next Launch
https://api.elonmu.sh/v1/launches/next?apikey=YOUR_API_KEY
Get Launch by Id
https://api.elonmu.sh/v1/launches/:id?apikey=YOUR_API_KEY
Get Company Info
https://api.elonmu.sh/v1/company?apikey=YOUR_API_KEY
Get Roadster Info
https://api.elonmu.sh/v1/roadster?apikey=YOUR_API_KEY
Authentication
Authentication is handled via API key. Each request that you make to the API must include your API key as a parameter in the request URL. Here's an example of how to make a request to get the latest launch data using JavaScript:
const apiKey = "YOUR_API_KEY";
fetch(`https://api.elonmu.sh/v1/launches/latest?apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data));
JavaScript Examples
Here are some more examples in JavaScript of how to use the Elon Mush API:
Get Latest Launch Data
const apiKey = "YOUR_API_KEY";
fetch(`https://api.elonmu.sh/v1/launches/latest?apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Get All Launches
const apiKey = "YOUR_API_KEY";
fetch(`https://api.elonmu.sh/v1/launches?apikey=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Search for Launches
const apiKey = "YOUR_API_KEY";
const query = "Falcon 9";
fetch(`https://api.elonmu.sh/v1/launches?apikey=${apiKey}&search=${query}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
Conclusion
The Elon Mush API is a powerful tool that provides access to a wealth of SpaceX data. With the examples provided in this guide, you should be well on your way to integrating the API into your own JavaScript applications. Happy coding!