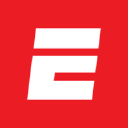
ESPN Sports API
Sports & FitnessUtilizing ESPN's API with JavaScript
If you're looking for sports statistics or information from ESPN's massive database, their public API is a great place to start. In this blog post, we'll go through how to use ESPN's API with JavaScript, using various examples.
First of all, we'll need to get an API key from ESPN's Developer Center. You can register for free and get a key in no time. Once you have the key, you'll be able to use any of the APIs described in http://www.espn.com/apis/devcenter/io-docs.html.
Making a Basic Request
Let's start with the simplest example. In this example, we'll get the full schedule of all the events for a specific league for a specific date. We will use the http
module to make a request to the API and use the JSON.parse()
method to parse the JSON data that we get back.
const http = require('http');
const apiKey = 'your-api-key-goes-here';
const league = 'nfl'; // This could also be nba, mlb, etc.
const date = '20190210'; // The date should be in yyyyMMdd format
const options = {
hostname: 'site.api.espn.com',
path: `/apis/site/v2/sports/football/${league}/scoreboard?dates=${date}&limit=1000&apikey=${apiKey}`,
method: 'GET'
};
const req = http.request(options, (res) => {
console.log(`Status Code: ${res.statusCode}`);
res.on('data', (d) => {
const parsedData = JSON.parse(d);
console.log(parsedData); // Log the response to the console
});
});
req.on('error', (error) => {
console.error(error);
});
req.end();
Accessing Specific Game Data
Now let's say we want to get even more specific and just see the information of a particular game. We can do that by utilizing the Game ID, which is specific to each game. Here's an example of how to retrieve the box score for a game using its ID:
const http = require('http');
const apiKey = 'your-api-key-goes-here';
const gameId = '400975325'; // Use the game ID provided by ESPN
const options = {
hostname: 'site.api.espn.com',
path: `/apis/site/v2/sports/football/nfl/summary?event=${gameId}&apikey=${apiKey}`,
method: 'GET'
};
const req = http.request(options, (res) => {
console.log(`Status Code: ${res.statusCode}`);
res.on('data', (d) => {
const parsedData = JSON.parse(d);
console.log(parsedData); // Log the response to the console
});
});
req.on('error', (error) => {
console.error(error);
});
req.end();
Implementing Error Handling
In the previous examples, if there was an error with the request or response, we simply logged it to the console. Let's take it a step further and handle the errors properly.
const http = require('http');
// API key and options stay the same as the previous examples
const req = http.request(options, (res) => {
if (res.statusCode < 200 || res.statusCode > 299) {
return console.log(`Error: Status Code ${res.statusCode}`);
}
let data = '';
res.on('data', (chunk) => {
data += chunk;
});
res.on('end', () => {
const parsedData = JSON.parse(data);
console.log(parsedData); // Log the response to the console
});
});
req.on('error', (error) => {
console.error(error);
});
req.end();
This implementation will ensure that we get the response data only if the status code falls within the 2xx range. If it falls outside that range, we log the error message to the console.
Conclusion
There you have it. This should be enough to get you started with using ESPN's public API with JavaScript. Depending on the type of data you're looking for, you might need to specify different options in your requests. When doing so, make sure to follow ESPN's documentation closely and adjust the examples to fit your needs. Happy coding!