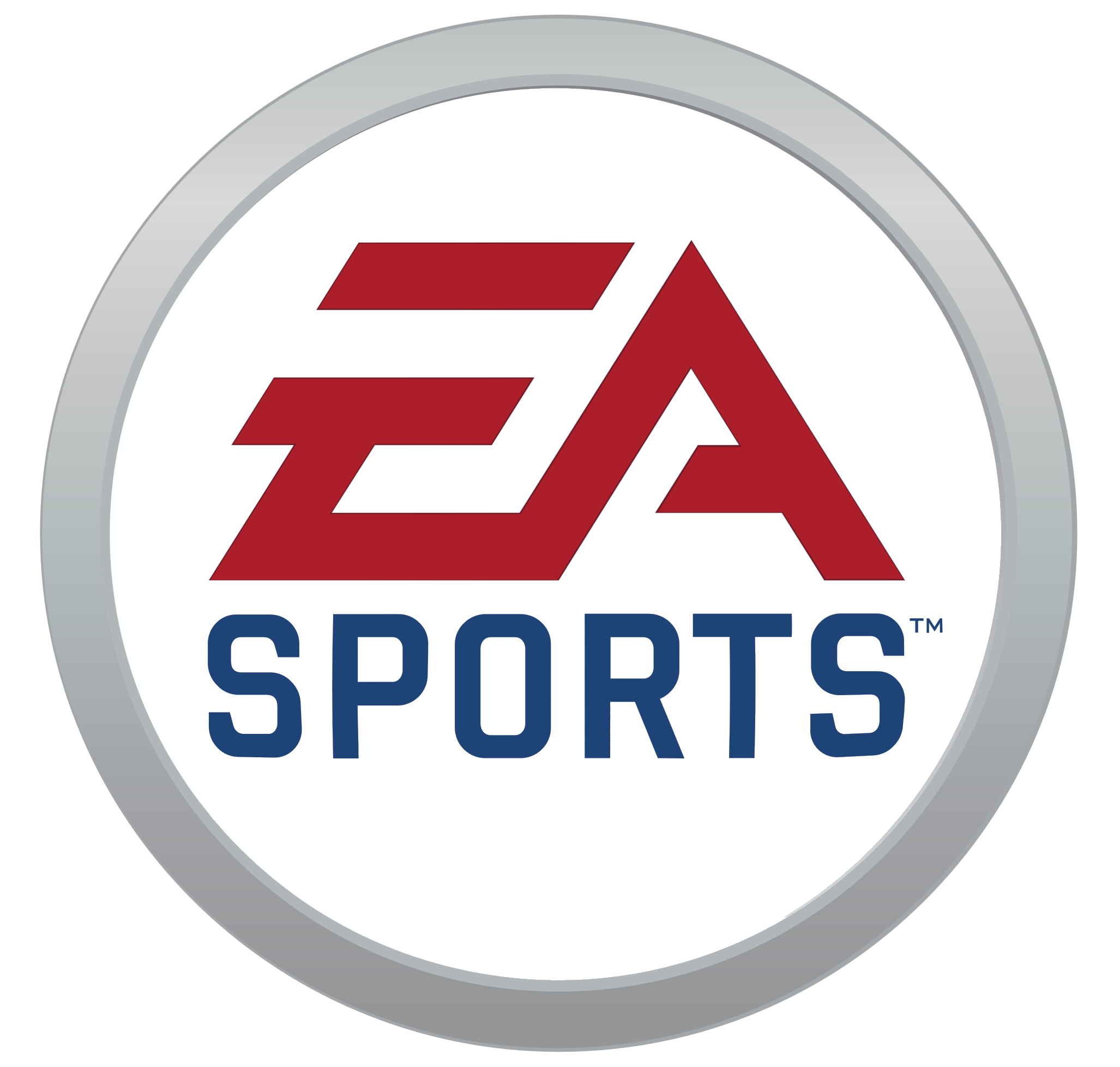
FIFA Ultimate Team
Sports & FitnessUsing EA Sports FIFA Ultimate Team API
EA Sports FIFA Ultimate Team API is a public API that provides access to various data related to FIFA 21 Ultimate Team game. The API provides access to Player, Club and League items including stats and attributes for each item. In this blog post, we'll explore how to use this API using JavaScript.
How to use FIFA Ultimate Team API
To use the FIFA Ultimate Team API, you first need to register with EA Developer Portal and request an API key. Once you have the API key, you can use it to access various endpoints and retrieve data.
API Endpoints
The FIFA Ultimate Team API includes a variety of endpoints to access different data and content types. This includes:
/fut/items
- Returns a list of player, club and league items./fut/item/{id}
- Returns a single player, club or league item by ID./fut/club/{id}
- Returns the club item of the specified ID./fut/league/{id}
- Returns the league item of the specified ID.
For this tutorial, we will be focusing on /fut/items
endpoint.
API Example in JavaScript
To use the API in JavaScript, you will first need to create a request to the appropriate API endpoint. You could use any Javascript library like axios
, fetch
or jQuery
. Here's an example using axios
library:
const axios = require('axios');
const apiKey = 'YOUR_API_KEY';
const url = 'https://www.easports.com/fifa/ultimate-team/api/fut/item';
axios.get(url, {
headers: { 'X-Auth-Token': apiKey }
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error.message);
});
In the above code, we imported axios
library and replaced YOUR_API_KEY
with your actual API key. We then set the url
to the FIFA Ultimate Team API endpoint and create a GET
request with the API key as a header. Finally, we log the response.data
to see the data returned from the endpoint.
Conclusion
In this guide, we learned how to use the EA Sports FIFA Ultimate Team API to retrieve player, club and league items data using JavaScript. While there are additional endpoints to explore with the API, the provided content should give you a good starting point for making calls and parsing data as necessary.