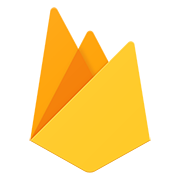
Face Detection with Firebase
Machine LearningWith ML Kit's face detection API, you can detect faces in an image, identify key facial features, and get the contours of detected faces. With face detection, you can get the information you need to perform tasks like embellishing selfies and portraits, or generating avatars from a user's photo. Because ML Kit can perform face detection in real time, you can use it in applications like video chat or games that respond to the player's expressions.
📚 Documentation & Examples
Everything you need to integrate with Face Detection with Firebase
🚀 Quick Start Examples
// Face Detection with Firebase API Example
const response = await fetch('https://firebase.google.com/docs/ml-kit/detect-faces', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using Firebase ML Kit to Detect Faces with JavaScript
Firebase ML Kit is a powerful tool that allows developers to add machine learning capabilities to their applications with ease. One of its features is the ability to detect faces in images using Artificial Intelligence.
In this blog post, we will go through the process of integrating Firebase ML Kit into a JavaScript application and detecting faces in an image.
Setting up Firebase ML Kit
First, we need to set up Firebase in our JavaScript project. Follow the steps below to get started:
- Create a Firebase project on the Firebase Console
- In the project settings, find the web app configuration and copy the Firebase config object
- Add the following code to your HTML file:
<!-- Add Firebase SDK -->
<script src="https://www.gstatic.com/firebasejs/8.6.5/firebase-app.js"></script>
<script src="https://www.gstatic.com/firebasejs/8.6.5/firebase-analytics.js"></script>
<!-- Initialize Firebase -->
<script>
var firebaseConfig = {
// Paste your Firebase config object here
};
firebase.initializeApp(firebaseConfig);
firebase.analytics();
</script>
Adding the Firebase ML Kit library
Next, we need to add the Firebase ML Kit library to our project. Add the following code to your HTML file:
<!-- Add Firebase ML Kit SDK -->
<script src="https://www.gstatic.com/firebasejs/8.6.5/firebase-ml.js"></script>
Detecting faces in an image
Now that we have set up Firebase ML Kit, we can start detecting faces in images. Add the following code to your JavaScript file:
// Get the image element
const img = document.getElementById('my-image');
// Get a reference to the Firebase ML vision instance
const vision = firebase.ml().vision();
// Create a new face detector instance
const faceDetector = vision.faceDetector();
// Detect faces in the image
faceDetector.process(img)
.then(faces => {
// Log the detected faces to the console
console.log(faces);
})
.catch(error => {
// Handle errors here
console.error(error);
});
In the code above, we first get a reference to the image element and the Firebase ML vision instance. We then create a new face detector instance and call the process
method to detect faces in the image. The then
method is called with an array of detected faces, which we log to the console.
Conclusion
Firebase ML Kit provides a simple and powerful way to add machine learning capabilities to JavaScript applications. With its face detection feature, you can easily detect faces in images and use that information in your application. We hope this blog post has been helpful in getting you started with Firebase ML Kit and face detection.