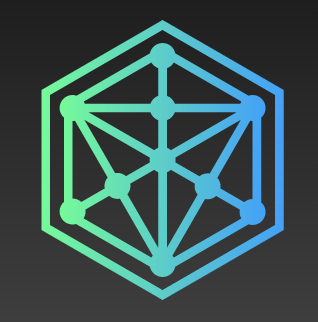
Faker API
Open DataFaker API it's a collection of completely free APIs that helps web developers and web designers generate fake data in a fast and easy way. No registration is required. No tokens, no authentication. Every resource allows to choose the API language by "_locale" parameter and also allows to select the number of rows requested by "_quantity" parameter. Max 1000 rows.
📚 Documentation & Examples
Everything you need to integrate with Faker API
🚀 Quick Start Examples
// Faker API API Example
const response = await fetch('https://fakerapi.it/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Using Faker API to Generate Fake Data
In today's evolving technological world, the use of API has become a popular trend. One of the most used APIs is the Faker API, which is a public API that allows developers to generate fake data such as names, addresses, and emails.
Faker API is easy to use and provides a wide range of fake data. It can be used to test different applications, websites, and software. In this article, we will show you how to use Faker API with JavaScript.
Getting Started with Faker API
To get started with Faker API, you need to sign up on the website, from where you can get your API key. Once you have your API key, you can start making requests to the API with the fake data you want to generate.
Faker API Example Code
Here are some examples of how you can use Faker API to generate fake data:
Generating a Fake Name
const url = `https://fakerapi.it/api/v1/persons?_quantity=1&gender=male`;
fetch(url, {
headers: {
'apikey': '<YOUR_API_KEY_HERE>',
},
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
Generating a Fake Address
const url = `https://fakerapi.it/api/v1/addresses?_quantity=1`;
fetch(url, {
headers: {
'apikey': '<YOUR_API_KEY_HERE>',
},
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
Generating a Fake Email
const url = `https://fakerapi.it/api/v1/internet?_quantity=1`;
fetch(url, {
headers: {
'apikey': '<YOUR_API_KEY_HERE>',
},
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
Generating a Fake Phone Number
const url = `https://fakerapi.it/api/v1/phones?_quantity=1`;
fetch(url, {
headers: {
'apikey': '<YOUR_API_KEY_HERE>',
},
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(error));
These are just a few examples of how you can use Faker API to generate fake data. There are many other fields you can use to generate fake data, such as date, city, company, and more.
Conclusion
Overall, Faker API is a useful tool that can help developers create realistic data for testing purposes. With this tutorial, you can now generate fake data using Faker API in your JavaScript projects. Enjoy!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes