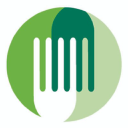
Food Standards Agency
GovernmentPublic Rest API Documentation for Food Hygiene Ratings
The Food Hygiene Ratings API provides publicly available data on food hygiene ratings and inspection outcomes for food businesses in the UK. The API allows developers to access food hygiene ratings data in a convenient, machine-readable format. In this blog, we will explore the API with some possible example code in JavaScript.
API Endpoint
The API endpoint for the Food Hygiene Ratings API is http://api.ratings.food.gov.uk/. The endpoint takes the form of a RESTful API, where developers can access data through several HTTP methods such as GET, POST, PUT, and DELETE. Each HTTP request method returns a different data response and status code.
API Example code in JavaScript
Getting information about a food business
To get information about a food business, you can query the API endpoint using the GET method with a URL parameter specifying the business ID. Here is an example of how to get the food hygiene rating for a business using JavaScript:
async function getFoodHygieneRating(businessId) {
const url = `http://api.ratings.food.gov.uk/businesses/${businessId}`;
const response = await fetch(url);
const data = await response.json();
return data.RatingValue;
}
getFoodHygieneRating(12345)
.then(rating => console.log(`Food Hygiene Rating: ${rating}`))
.catch(err => console.error(err));
Searching for food businesses
To search for food businesses, you can query the API endpoint using the GET method with a URL parameter specifying the search term. Here is an example of how to search for food businesses by name using JavaScript:
async function searchFoodBusiness(name) {
const url = `http://api.ratings.food.gov.uk/businesses?q=${name}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
searchFoodBusiness('Pizza Hut')
.then(businesses => console.log(`Found ${businesses.length} businesses`))
.catch(err => console.error(err));
Retrieving food hygiene ratings
To retrieve food hygiene ratings, you can query the API endpoint using the GET method with a URL parameter specifying the authority ID and rating value. Here is an example of how to retrieve food hygiene ratings using JavaScript:
async function getRatings(authorityId, rating) {
const url = `http://api.ratings.food.gov.uk/ratings?localAuthorityId=${authorityId}&ratingKey=${rating}`;
const response = await fetch(url);
const data = await response.json();
return data;
}
getRatings('927', '5')
.then(ratings => console.log(`Found ${ratings.length} businesses with rating of 5`))
.catch(err => console.error(err));
Conclusion
The Food Hygiene Ratings API is an important public resource for developers looking to access information on food businesses in the UK. With the above example code in JavaScript, you can quickly query the API to retrieve rating information and search for food businesses.