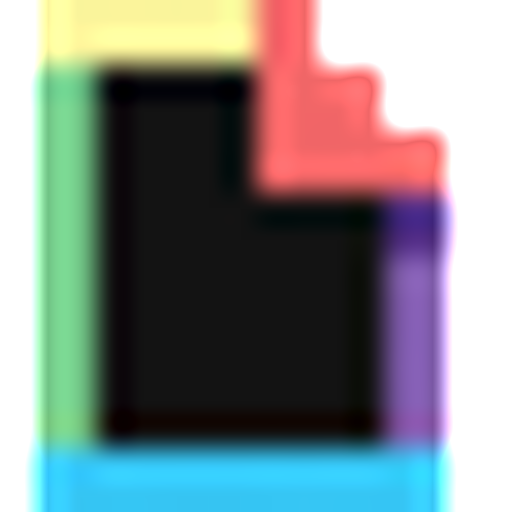
Giphy
PhotographyExploring GIPHY API with JavaScript
Are you a developer looking to add some fun to your project? The GIPHY API offers a way to search for and display animated GIFs on your website or application. In this article, we will be exploring the various endpoints of the GIPHY API and how to use them with JavaScript.
Getting Started
To get started, you first need to sign up for a GIPHY API key here. Once you have your API key, you can start making requests to the GIPHY API.
Search for GIFs
To search for GIFs using the GIPHY API, we can use the search
endpoint. Here's an example of how to use this endpoint with JavaScript:
const apiKey = 'YOUR_API_KEY';
const searchQuery = 'puppies';
const searchEndpoint = `https://api.giphy.com/v1/gifs/search?api_key=${apiKey}&q=${searchQuery}`;
fetch(searchEndpoint)
.then(response => response.json())
.then(data => console.log(data));
In the above code, we are making a fetch
request to the search
endpoint with the search query puppies
. We are also passing in our API key as a query parameter.
This will return a JSON object with an array of GIFs that match our search query.
Displaying GIFs
Once we have fetched our GIFs, we can display them on our website using the img
element in HTML. Here's an example of how to display a single GIF:
<img src="https://media.giphy.com/media/3o85xwx9Nv2hbCp9Qc/giphy.gif" />
In the above code, we are displaying a single GIF with a src
attribute that points to the GIF's URL.
If we want to display multiple GIFs, we can loop through the array of results and create an img
element for each one. Here's an example:
const apiKey = 'YOUR_API_KEY';
const searchQuery = 'puppies';
const searchEndpoint = `https://api.giphy.com/v1/gifs/search?api_key=${apiKey}&q=${searchQuery}`;
fetch(searchEndpoint)
.then(response => response.json())
.then(data => {
const gifs = data.data;
const container = document.getElementById('gifs');
gifs.forEach(gif => {
const img = document.createElement('img');
img.src = gif.images.fixed_width.url;
container.appendChild(img);
});
});
In the above code, we are creating an img
element for each GIF in the search results and adding it to a container element on our website.
Conclusion
In this article, we explored the GIPHY API and how to use it with JavaScript. We learned how to search for GIFs and display them on our website. With the GIPHY API, you can add a touch of humor and fun to your projects. Happy coding!