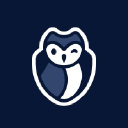
GitGuardian
SecurityThe API designed for scanning files for secrets, such as API keys and database credentials, is an essential tool for developers and security teams looking to safeguard sensitive information. By integrating this API into your workflow, you can automate the detection of hard-coded secrets in your codebase, preventing potential data breaches and ensuring compliance with best security practices. This proactive measure not only enhances the security posture of your applications but also fosters trust with your users by demonstrating a commitment to safeguarding their data.
Utilizing this API provides multiple benefits that can help streamline your development process and enhance security protocols. Key advantages include:
- Automated Scanning: Automatically detect sensitive information in code to eliminate human error.
- Real-time Alerts: Receive immediate notifications about potential exposure of secrets across your repositories.
- Comprehensive Reporting: Access detailed reports that outline detected secrets and provide recommendations for remediation.
- Flexible Integration: Easily integrate the API into existing CI/CD pipelines to ensure continuous security checks.
- Secure Development Practices: Enhance team awareness and adherence to coding standards that prevent leaks of sensitive data.
Here’s a JavaScript code example demonstrating how to call the API:
const axios = require('axios');
const scanFileForSecrets = async (fileContent) => {
try {
const response = await axios.post('https://api.gitguardian.com/scans', {
file: fileContent,
}, {
headers: {
'Authorization': `Bearer YOUR_API_TOKEN`,
'Content-Type': 'application/json'
}
});
console.log('Scan Results:', response.data);
} catch (error) {
console.error('Error scanning file:', error.response ? error.response.data : error.message);
}
};
// Example usage
const fileContent = "Your file content with potential secrets";
scanFileForSecrets(fileContent);