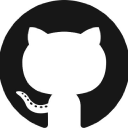
Github Jobs
JobsJobs for software developers. GitHub Jobs is a great place attract the best technical talent for your company's open software development positions. The GitHub Jobs API allows you to search, and view jobs with JSON over HTTP. To get the JSON representation of any search result or job listing, append .json to the URL you'd use on the HTML GitHub Jobs site.
📚 Documentation & Examples
Everything you need to integrate with Github Jobs
🚀 Quick Start Examples
// Github Jobs API Example
const response = await fetch('https://jobs.github.com/api', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Exploring the GitHub Jobs API with JavaScript
If you're a developer searching for employment, GitHub Jobs can be an excellent resource. They provide a useful API for accessing job postings. In this tutorial, we'll explore querying their API with JavaScript.
Getting Started
The first step is to get your hands on an API key. Navigate to the API documentation and click "Get API key." After signing in, you'll be able to access the API key.
API Endpoints
The GitHub Jobs API has two primary endpoints.
Job Search Endpoint
The first is a job search endpoint. Here's an example of how to access it using JavaScript:
let url = 'https://jobs.github.com/positions.json'
let data = {
description: 'javascript',
location: 'san francisco',
}
let params = Object.keys(data).map(key => key + '=' + encodeURIComponent(data[key])).join('&');
let fullUrl = `${url}?${params}`;
fetch(fullUrl)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code searches for JavaScript jobs in San Francisco.
Job Endpoint
The second endpoint returns a detailed job listing. Here's how to use it:
let jobId = '1d8c72ef-807f-4646-bb6d-fd5c7d157051'; // Job ID
let url = `https://jobs.github.com/positions/${jobId}.json`;
fetch(url)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This code retrieves data for a specific job with the given ID.
Conclusion
The GitHub Jobs API is an amazing resource for anyone searching for employment. It can be used with any language, and, as demonstrated here, it's easy to use with JavaScript. So explore the API endpoints and find the job of your dreams!
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes