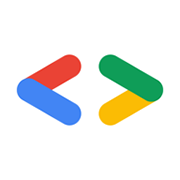
Google Safe Browsing
Anti-MalwareAn introduction to Google Safe Browsing API
What is Google Safe Browsing API?
Google Safe Browsing API is a public API offered by Google that helps developers to protect their applications and websites from malware, phishing and other deceptive content on the web.
Developers can integrate this API to their applications and web services to enable safe browsing features to prevent users from accessing harmful content on the internet.
In this article, we will explore different commonly used APIs of Google Safe Browsing API along with code implementation in JavaScript.
Features of Google Safe Browsing API
Google Safe Browsing API offers the following features-
-
Malware scanning: Scan and detect malware on a webpage or website.
-
Phishing protection: Detect phishing attempts and warn users, prevent them from sharing their sensitive information with phishing sites.
-
Suspicious download protection: Prevent users from downloading harmful files from unsafe sources.
How to use Google Safe Browsing API
Before using Google Safe Browsing API, you need to get an API key from Google. You can get an API key by creating a project in the Google Cloud Console and enable Safe Browsing API in your project.
Once you have the API key, you can use any one of the following APIs in your application.
Lookup API
This API allows you to check whether a webpage or website is considered safe or dangerous by Google. The API takes a URL as an input and returns a response as either safe or unsafe along with other details.
fetch('https://safebrowsing.googleapis.com/v4/threatMatches:find?key=YOUR_API_KEY', {
method: 'POST',
body: JSON.stringify({
"client": {
"clientId": "yourcompanyname",
"clientVersion": "1.5.2"
},
"threatInfo": {
"threatTypes": ["MALWARE", "SOCIAL_ENGINEERING"],
"platformTypes": ["WINDOWS"],
"threatEntryTypes": ["URL"],
"threatEntries": [
{"url": "http://www.urltocheck1.com/"},
{"url": "http://www.urltocheck2.com/"}
]
}
}),
}).then(response => {
console.log(response)
}).catch(err => {
console.log('Error: ', err)
})
GetFullHashes
This API is used to retrieve the SHA256 hashes of all known safe and unsafe URLs, which can be used to improve the detection of potential threats in your application.
fetch('https://safebrowsing.googleapis.com/v4/fullHashes:find?key=YOUR_API_KEY', {
method: 'POST',
body: JSON.stringify({
"client": {
"clientId": "yourcompanyname",
"clientVersion": "1.5.2"
},
"threatInfo": {
"threatTypes": ["MALWARE", "SOCIAL_ENGINEERING"],
"platformTypes": ["WINDOWS"],
"threatEntryTypes": ["URL"],
"threatEntries": [
{"url": "http://www.urltocheck1.com/"},
{"url": "http://www.urltocheck2.com/"}
]
}
}),
}).then(response => {
console.log(response)
}).catch(err => {
console.log('Error: ', err)
})
Update API
This API is used to update the local safe browsing database of your application with the latest version of Google Safe Browsing API.
fetch('https://safebrowsing.googleapis.com/v4/threatListUpdates:fetch?key=YOUR_API_KEY', {
method: 'POST',
body: JSON.stringify({
"client": {
"clientId": "yourcompanyname",
"clientVersion": "1.5.2"
},
"listUpdateRequests": [
{
"threatType": "MALWARE",
"platformType": "WINDOWS",
"threatEntryType": "URL",
"state": {
"threatEntries": [
{"url": "http://www.urltocheck1.com/"},
{"url": "http://www.urltocheck2.com/"}
]
}
}
]
}),
}).then(response => {
console.log(response)
}).catch(err => {
console.log('Error: ', err)
})
In conclusion, the Google Safe Browsing API provides an added layer of security against malware, phishing attacks and other deceptive content on the web. By integrating the above-mentioned APIs, you can protect your application and website from potential threats and ensure the safety of your users.