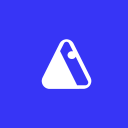
GraphQL Jobs
JobsIntroduction to the graphql.jobs Public API
graphql.jobs is a public API that provides access to a database of job listings related to GraphQL technology. This API allows users to search for job listings using various queries such as job title, company name, and location. In this blog post, we will explore some examples of how to use the graphql.jobs API with JavaScript.
Getting Started
Firstly, you will need to obtain an API key from the Graphql.jobs website. With this key, you will be able to make requests to the API. Once you have your API key, you can make requests using the endpoints provided by the API.
Example Requests
In the following examples, we will use the popular Axios library in JavaScript to make requests to the graphql.jobs API.
Query for Job Listings
const axios = require('axios');
const API_URL = 'https://api.graphql.jobs/';
const query = `
query {
jobs {
id
title
company {
name
}
}
}
`;
const config = {
headers: {
Authorization: `Bearer YOUR_API_KEY`,
},
};
axios.post(API_URL, { query }, config)
.then((res) => {
console.log(res.data.data.jobs);
})
.catch((error) => {
console.log(error);
});
The above code sends a query to retrieve all job listings from the graphql.jobs API. It specifies that the returned data should include the job ID, job title, and the name of the company. The response data is logged to the console.
Search for Job Listings by Title and Location
const axios = require('axios');
const API_URL = 'https://api.graphql.jobs/';
const query = `
query {
jobs(title: "frontend", location: "new york") {
id
title
company {
name
}
}
}
`;
const config = {
headers: {
Authorization: `Bearer YOUR_API_KEY`,
},
};
axios.post(API_URL, { query }, config)
.then((res) => {
console.log(res.data.data.jobs);
})
.catch((error) => {
console.log(error);
});
This code sends a query to retrieve all job listings that contain the word "frontend" in the job title and are located in "new york". The returned data includes the same fields as in the previous example.
Search for Job Listings by Company
const axios = require('axios');
const API_URL = 'https://api.graphql.jobs/';
const query = `
query {
jobs(companyName: "facebook") {
id
title
company {
name
}
}
}
`;
const config = {
headers: {
Authorization: `Bearer YOUR_API_KEY`,
},
};
axios.post(API_URL, { query }, config)
.then((res) => {
console.log(res.data.data.jobs);
})
.catch((error) => {
console.log(error);
});
This code searches for job listings by company name. It retrieves all job listings with the company name "facebook". The returned data includes the same fields as in the previous examples.
Conclusion
In this blog post, we explored some examples of how to use the graphql.jobs public API with JavaScript and Axios. With this API, users have access to job listings related to GraphQL technology and can search for job listings using various queries.
We recommend checking out the graphql.jobs documentation for more information on the API's endpoints and query types. Happy coding!