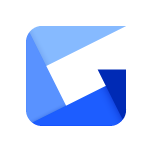
Gyazo
PhotographyGyazo API: A Comprehensive Guide
Gyazo API is a powerful tool that allows you to integrate Gyazo screenshots and captures within your applications. With the use of Gyazo API, you can easily capture screenshots, share them online, and embed them in your web pages. In this article, we will give you a step-by-step guide on how to use Gyazo API with examples in JavaScript.
Getting Started
To use Gyazo API, you need to have an API token. You can get your token from Gyazo API Key page. After obtaining the access token, you can start using the Gyazo API.
Uploading Images
The simplest way to upload an image to Gyazo is to use the gyazo.com/api/upload
endpoint. You can pass the image data as a base64-encoded string in the request body. The response will contain the image URL.
const image = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAAUAAAAFCAYAAACNbyblAAAAHElEQVQI12P4//8/w38GIAXDIBKE0DHxgljNBAAO9TXL0Y4OHwAAAABJRU5ErkJggg==';
const response = await fetch('https://upload.gyazo.com/api/upload', {
method: 'POST',
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/json',
},
body: JSON.stringify({
imagedata: image,
}),
});
const data = await response.json();
console.log(data.url);
Capturing Screenshots
You can also use Gyazo API to capture screenshots. The gyazo.com/api/capture
endpoint is used for capturing screenshots. When you call this endpoint, Gyazo creates a screenshot of the specified area of your screen.
const response = await fetch('https://upload.gyazo.com/api/capture', {
method: 'POST',
headers: {
Authorization: `Bearer ${accessToken}`,
},
});
const data = await response.json();
console.log(data.url);
Updating Image Properties
You can use the gyazo.com/api/images/:id
endpoint to update properties of an image. For example, you can update the title and description of an image using this endpoint.
const imageId = 'sampleImageId';
const newTitle = 'New Title';
const newDescription = 'New Description';
const response = await fetch(`https://api.gyazo.com/api/images/${imageId}`, {
method: 'PUT',
headers: {
Authorization: `Bearer ${accessToken}`,
'Content-Type': 'application/json',
},
body: JSON.stringify({
title: newTitle,
description: newDescription,
}),
});
const data = await response.json();
console.log(data);
Deleting Images
You can use the gyazo.com/api/images/:id
endpoint to delete an image.
const imageId = 'sampleImageId';
const response = await fetch(`https://api.gyazo.com/api/images/${imageId}`, {
method: 'DELETE',
headers: {
Authorization: `Bearer ${accessToken}`,
},
});
console.log(`Image ${imageId} has been deleted`);
Conclusion
In this article, we have shown you the basics of using Gyazo API with examples in JavaScript. With this information, you can easily integrate Gyazo screenshots and captures into your applications. You can find more information about Gyazo API on Gyazo API Docs.