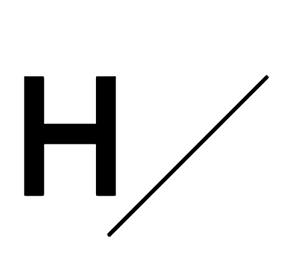
Harvard Art Museums
Art & DesignAccessing Harvard Art Museums Collections using Public API
Harvard Art Museums provides a public API that allows users to access its vast collection data. This collection includes artworks, photographs, archival materials, and much more. This API is a great resource for researchers, educators, and students alike. In this blog, we will explore how to access the Harvard Art Museums Collections using the Public API.
Getting Started
To use the API, first, you have to obtain an API key. You can obtain the key by registering on the Harvard Art Museums API website. Once you obtain your API key, you can start making API requests.
Making API Requests
All API requests are sent as HTTPS GET requests, and the response is in JSON format. In the following example codes, we will use JavaScript to make API requests to the Harvard Art Museums Collection API.
Example 1 - Search for Objects by Keyword:
This API endpoint allows users to search the collection by keyword.
const url = 'https://api.harvardartmuseums.org/object';
const queryParams = {
apikey: 'yourapikeyhere',
keyword: 'pottery',
};
const searchUrl = new URLSearchParams(queryParams);
fetch(`${url}?${searchUrl}`)
.then(response => response.json())
.then(data => console.log(data));
Example 2 - Retrieve an Artwork by Id:
This API endpoint allows users to retrieve an artwork by its id.
const url = `https://api.harvardartmuseums.org/object/:id`;
const queryParams = {
apikey: 'yourapikeyhere',
};
const searchUrl = new URLSearchParams(queryParams);
fetch(`${url}?${searchUrl}`)
.then(response => response.json())
.then(data => console.log(data));
Example 3 - Search for Users:
This API endpoint allows users to search the users associated with the collection.
const url = 'https://api.harvardartmuseums.org/person';
const queryParams = {
apikey: 'yourapikeyhere',
q: 'john',
};
const searchUrl = new URLSearchParams(queryParams);
fetch(`${url}?${searchUrl}`)
.then(response => response.json())
.then(data => console.log(data));
Example 4 - Search for Galleries:
This API endpoint allows users to search for galleries associated with the collection.
const url = 'https://api.harvardartmuseums.org/gallery';
const queryParams = {
apikey: 'yourapikeyhere',
q: 'italian',
};
const searchUrl = new URLSearchParams(queryParams);
fetch(`${url}?${searchUrl}`)
.then(response => response.json())
.then(data => console.log(data));
Example 5 - Retrieve Metadata:
This API endpoint allows users to retrieve the collection metadata.
const url = 'https://api.harvardartmuseums.org/metadata';
const queryParams = {
apikey: 'yourapikeyhere',
};
const searchUrl = new URLSearchParams(queryParams);
fetch(`${url}?${searchUrl}`)
.then(response => response.json())
.then(data => console.log(data));
Conclusion
The Harvard Art Museums Collection API is a fantastic resource for researchers, educators, and students. With a simple API request, users can retrieve vast amounts of information in JSON format. This blog highlights just a few of the API endpoints available in the collection API. With access to this API, users can retrieve a wealth of information about the artworks, photographs, archival materials, and much more.