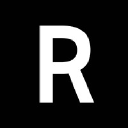
Rijksmuseum
Art & DesignThe RijksMuseum Data API offers an extensive collection of art-related metadata, providing developers and researchers access to the rich heritage of the Rijksmuseum's vast art collection. With the API, users can retrieve detailed information about artworks, artists, exhibitions, and more, allowing for innovative applications that can enhance digital experiences in education, curation, and creative projects. This resource is particularly valuable for web developers, academic institutions, and cultural organizations looking to integrate historical art data into their platforms or to create unique visualizations that celebrate Dutch art history.
Utilizing the RijksMuseum Data API provides several key benefits:
- Access to a comprehensive dataset from one of the most prestigious art museums in the world.
- Real-time updates and fresh content to ensure the latest information is readily available.
- A straightforward RESTful interface that simplifies integration into web and mobile applications.
- Support for diverse formats, including JSON, making it user-friendly for various programming languages.
- Opportunities for educational projects and cultural initiatives that promote engagement with art and history.
Here is a simple JavaScript code example for calling the RijksMuseum Data API:
fetch('https://www.rijksmuseum.nl/api/en/collection?key=YOUR_API_KEY&q=rembrandt')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('Error fetching data:', error);
});