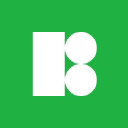
Icons8
Art & DesignThe Icons API from Icons8 is a powerful tool for developers and designers seeking high-quality icons for their applications or websites. With a vast collection of icons covering various categories, users can easily find and implement visually appealing graphics to enhance user experience. The API allows for seamless integration, enabling you to dynamically retrieve icons based on your specifications. For more information and detailed documentation, visit Icons8 documentation. The API supports a straightforward search functionality, meaning you can easily locate icons like the "search icon" hyperlink directly on the page, improving the efficiency of your workflow.
Utilizing the Icons API presents numerous advantages that can elevate your project’s design and functionality. Here are some key benefits:
- Access to a wide variety of customizable icons and graphics.
- Simple and intuitive API structure for ease of integration.
- High-resolution icons suitable for both web and mobile applications.
- Regularly updated library ensures fresh and modern designs.
- Extensive documentation and community support for troubleshooting.
Here is a JavaScript code example demonstrating how to call the Icons API to fetch a search icon:
fetch('https://api.icons8.com/icons8/v1/icons/media/search')
.then(response => response.json())
.then(data => {
const searchIconUrl = data.url;
console.log('Search Icon URL:', searchIconUrl);
})
.catch(error => {
console.error('Error fetching the icon:', error);
});