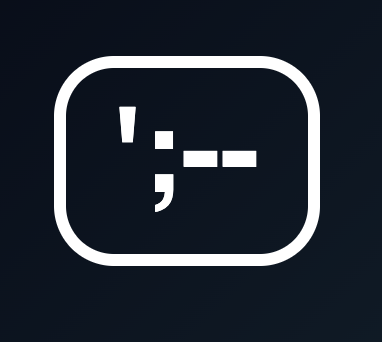
HaveIBeenPwned
SecurityHow to Use the Have I Been Pwned API with JavaScript
Have I Been Pwned is a free website that allows users to check if their personal information has been compromised in a data breach. The site provides an API that developers can use to integrate the data into their own applications. In this blog post, we'll cover how to use the Have I Been Pwned API with JavaScript.
Getting Started
Before we dive into the code, we need to sign up for a Have I Been Pwned API key. You can do this by visiting the API website - https://haveibeenpwned.com/API/v2 - and following the instructions. Once you have a key, you're ready to start making requests.
Examples
We'll start with a basic example to check if an email has been pwned:
const email = 'example@domain.com';
const apiKey = 'YOUR_API_KEY';
fetch(`https://haveibeenpwned.com/api/v2/breachedaccount/${email}?api_key=${apiKey}`)
.then(response => {
if (response.ok) {
console.log(`${email} has been pwned!`);
} else {
console.log(`${email} has not been pwned.`);
}
})
.catch(error => console.error(error));
In this example, we're using the Fetch API to send a GET request to the Have I Been Pwned API. We're passing the email address as a parameter in the URL, and we're also including our API key. The response will either be a list of breaches that the email was involved in, or it will be an empty array if the email hasn't been pwned.
Next, let's search for breaches by domain:
const domain = 'domain.com';
const apiKey = 'YOUR_API_KEY';
fetch(`https://haveibeenpwned.com/api/v2/breaches?domain=${domain}&api_key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
This example sends a GET request to the /breaches endpoint with the domain parameter. We're also passing our API key. The response will be a list of breaches that have affected the specified domain.
Finally, let's search for a breach by name:
const name = 'Adobe';
const apiKey = 'YOUR_API_KEY';
fetch(`https://haveibeenpwned.com/api/v2/breach/${name}?api_key=${apiKey}`)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
In this example, we're using the /breach endpoint to search for a specific breach by name. The response will be the details of the specified breach.
Conclusion
That's it! With these examples, you can start using the Have I Been Pwned API to check for data breaches in your own applications. Remember to always keep user privacy in mind and handle their data with care.