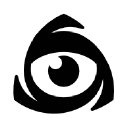
Iconfinder
Art & DesignAccessing Iconfinder API with JavaScript
If you're a developer looking to access the Iconfinder API using JavaScript, you've come to the right place! In this blog post, we'll go through some examples of how to make API calls using JavaScript.
Getting Started
To access the Iconfinder API, you'll first need to create an account on their developer website and get an API key. Once you have your API key, you can start making API requests.
Endpoints
Iconfinder API has several endpoints you can use to get different types of data. Here are some examples:
Search icons
You can search for icons by using the /v4/icons/search
endpoint. Here is an example code snippet:
let query = "computer";
let url = "https://api.iconfinder.com/v4/icons/search?query=" + query;
let options = {
method: "GET",
headers: {
Authorization: "Bearer YOUR_API_KEY_GOES_HERE",
},
};
fetch(url, options)
.then((response) => response.json())
.then((data) => console.log(data));
Replace YOUR_API_KEY_GOES_HERE
with your actual API key.
Get icon details
To get details about an icon, use the /v4/icons/{icon_id}
endpoint. Here is an example code snippet:
let icon_id = "123456789";
let url = `https://api.iconfinder.com/v4/icons/${icon_id}`;
let options = {
method: "GET",
headers: {
Authorization: "Bearer YOUR_API_KEY_GOES_HERE",
},
};
fetch(url, options)
.then((response) => response.json())
.then((data) => console.log(data));
Again, replace YOUR_API_KEY_GOES_HERE
with your actual API key and 123456789
with a valid icon ID.
Get icon sets
You can also get icon sets using the /v4/iconsets
endpoint. Here is an example code snippet:
let url = "https://api.iconfinder.com/v4/iconsets";
let options = {
method: "GET",
headers: {
Authorization: "Bearer YOUR_API_KEY_GOES_HERE",
},
};
fetch(url, options)
.then((response) => response.json())
.then((data) => console.log(data));
Replace YOUR_API_KEY_GOES_HERE
with your actual API key.
Conclusion
In this blog post, we've gone over some examples of how to use the Iconfinder API with JavaScript. To get started with your own application, head over to their developer website and start experimenting with the API!