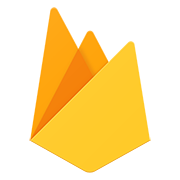
Image Labeling
Machine LearningFirebase ML Kit - Label Images API Examples in JavaScript
Firebase ML Kit is a powerful tool for machine learning, allowing developers to easily integrate machine learning technologies into their applications. With ML Kit, developers can train models to detect and recognize objects in images, recognize text in images, and many other tasks. In this blog post, we will explore the Label Images API of ML Kit and provide some JavaScript code examples for using it.
Label Images API Overview
The Label Images API is part of Firebase ML Kit's image recognition capabilities. It allows developers to detect and classify objects within an image. Once an object is detected, the API provides information about the object, such as type and the location within the image.
The Label Images API is powered by Google Cloud Vision, which provides machine learning models that have been trained on millions of images. As a result, the Label Images API is able to detect and classify a wide range of objects with high accuracy.
Code Examples
Initializing Firebase
To use the Label Images API, you will need to initialize Firebase in your JavaScript project. Here's an example of how to do that:
const firebaseConfig = {
// Your Firebase configuration goes here
};
firebase.initializeApp(firebaseConfig);
Loading an Image
Before you can use the Label Images API to classify an image, you will need to load the image into your project. Here's an example of how to do that using the HTML5 File API:
const fileInput = document.querySelector('input[type="file"]');
const img = new Image();
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0];
const reader = new FileReader();
reader.onload = (readerEvent) => {
img.src = readerEvent.target.result;
};
reader.readAsDataURL(file);
});
Detecting Labels in an Image
Once you have loaded an image into your project, you can use the Label Images API to detect and classify objects within the image. Here's an example of how to do that:
const vision = firebase.vision();
const image = new FirebaseVisionImageFromImage(img);
const labeler = vision.cloudImageLabeler();
labeler.processImage(image)
.then((labels) => {
labels.forEach((label) => {
// Do something with the label data
});
})
.catch((error) => {
console.error(error);
});
In this example, we first create a FirebaseVisionImage object from the loaded image, and then create a CloudImageLabeler using Firebase ML Kit's vision() method. We then pass the image to the labeler's processImage() method, which returns a Promise that resolves to an array of Label objects. We can then iterate over the array of Label objects to access information about each detected object.
Conclusion
In this blog post, we explored the Label Images API of Firebase ML Kit and provided some code examples for using it in a JavaScript project. With the Label Images API, developers can easily detect and classify objects within an image, making it a powerful tool for machine learning applications.