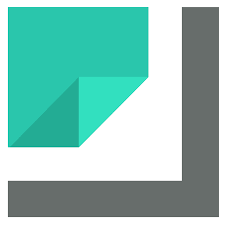
Imagga
Machine LearningImagga is a website that offers image recognition solutions for businesses and individuals. Their services include tagging, visual search, and NSFW moderation, which can help improve organization and searchability of images, streamline image-based workflows, and ensure that inappropriate content is kept off your platform. With advanced algorithms and machine learning capabilities, Imagga is able to accurately analyze and categorize images, providing reliable results and efficient solutions. Whether you're a photographer, marketer, or content creator, Imagga's image recognition tools can help you save time and improve the quality of your work. Try out their services today and experience the benefits of advanced image recognition technology.
📚 Documentation & Examples
Everything you need to integrate with Imagga
🚀 Quick Start Examples
// Imagga API Example
const response = await fetch('https://imagga.com/', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
});
const data = await response.json();
console.log(data);
Introduction
Have you ever wondered how some websites are able to automatically tag and categorize images? The answer lies in the use of APIs such as the one provided by Imagga. In this blog post, we will explore the Imagga API and provide examples of how to integrate it into your applications using JavaScript.
The Imagga API
The Imagga API provides access to powerful image recognition algorithms that can be used to automatically categorize and tag images. The API offers a range of features, including:
- Color extraction
- Object recognition
- Image categorization
- Tagging
Getting Started with the Imagga API
To use the Imagga API, you will need to sign up for an account and obtain an API key. Once you have your API key, you can start making requests to the API using JavaScript.
Color Extraction
To extract colors from an image using the Imagga API, you can use the following code:
const API_KEY = 'YOUR_API_KEY';
const imageFile = 'PATH_TO_IMAGE_FILE';
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://api.imagga.com/v2/colors', true);
xhr.setRequestHeader('Authorization', `Basic ${btoa(API_KEY)}`);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response.result.colors);
}
};
const formData = new FormData();
formData.append('image', imageFile);
xhr.send(formData);
This will extract the dominant colors from the image and return them as an array of objects. Each object will contain the color code, percentage, and other data.
Object Recognition
To recognize objects in an image using the Imagga API, you can use the following code:
const API_KEY = 'YOUR_API_KEY';
const imageFile = 'PATH_TO_IMAGE_FILE';
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://api.imagga.com/v2/categories/personal_photos', true);
xhr.setRequestHeader('Authorization', `Basic ${btoa(API_KEY)}`);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response.result);
}
};
const formData = new FormData();
formData.append('image', imageFile);
xhr.send(formData);
This will recognize the objects in the image and return them as a list of categories.
Image Categorization
To categorize an image using the Imagga API, you can use the following code:
const API_KEY = 'YOUR_API_KEY';
const imageFile = 'PATH_TO_IMAGE_FILE';
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://api.imagga.com/v2/categories/personal_photos', true);
xhr.setRequestHeader('Authorization', `Basic ${btoa(API_KEY)}`);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response.result.categories);
}
};
const formData = new FormData();
formData.append('image', imageFile);
xhr.send(formData);
This will categorize the image and return the top categories for the image.
Tagging
To tag an image using the Imagga API, you can use the following code:
const API_KEY = 'YOUR_API_KEY';
const imageFile = 'PATH_TO_IMAGE_FILE';
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://api.imagga.com/v2/tags', true);
xhr.setRequestHeader('Authorization', `Basic ${btoa(API_KEY)}`);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
const response = JSON.parse(xhr.responseText);
console.log(response.result.tags);
}
};
const formData = new FormData();
formData.append('image', imageFile);
xhr.send(formData);
This will tag the image and return a list of tags associated with the image.
Conclusion
In this blog post, we explored the Imagga API and provided examples of how to integrate it into your applications using JavaScript. We looked at how to extract colors, recognize objects, categorize images, and tag images using the API. The Imagga API is a powerful tool that can help you automate the process of tagging and categorizing images, making it an essential component of many web applications.
📊 30-Day Uptime History
Daily uptime tracking showing online vs offline minutes